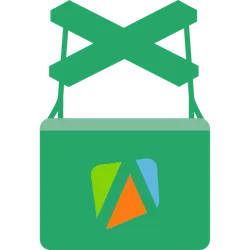
Example Puppeteer
Pricing
Pay per usage
Go to Store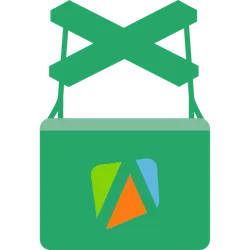
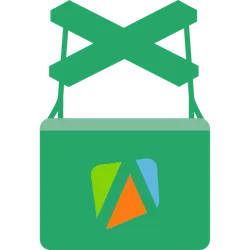
Example Puppeteer
Example showing how to use headless Chromium with Puppeteer to open a web page, determine its dimensions, save a screenshot, and print the page to PDF. This actor must use images with Puppeteer (Node.js 8 + Puppeteer on Debian).
4.6 (5)
Pricing
Pay per usage
7
Total users
390
Monthly users
12
Runs succeeded
>99%
Last modified
a year ago
Dockerfile
# This is a template for a Dockerfile used to run acts in Actor system.# The base image name below is set during the act build, based on user settings.# IMPORTANT: The base image must set a correct working directory, such as /usr/src/app or /home/userFROM apify/actor-node-puppeteer-chrome:15-10.1.0
# Second, copy just package.json and package-lock.json since it should be# the only file that affects "npm install" in the next step, to speed up the buildCOPY package*.json ./
# Install NPM packages, skip optional and development dependencies to# keep the image small. Avoid logging too much and print the dependency# tree for debuggingRUN npm --quiet set progress=false \ && npm install --only=prod --no-optional \ && echo "Installed NPM packages:" \ && (npm list --all || true) \ && echo "Node.js version:" \ && node --version \ && echo "NPM version:" \ && npm --version
# Copy source code to container# Do this in the last step, to have fast build if only the source code changedCOPY . ./
# NOTE: The CMD is already defined by the base image.# Uncomment this for local node inspector debugging:# CMD [ "node", "--inspect=0.0.0.0:9229", "main.js" ]
main.js
1const Apify = require('apify');2
3Apify.main(async () => {4 const input = await Apify.getValue('INPUT');5 6 if (!input || !input.url) throw new Error('Invalid input, must be a JSON object with the "url" field!');7 8 console.log('Launching Puppeteer...');9 const browser = await Apify.launchPuppeteer();10 11 console.log(`Opening URL: ${input.url}`); 12 const page = await browser.newPage();13 await page.goto(input.url);14 15 // Get the "viewport" of the page, as reported by the page.16 console.log('Determining page dimensions...');17 const dimensions = await page.evaluate(() => ({18 width: document.documentElement.clientWidth,19 height: document.documentElement.clientHeight,20 deviceScaleFactor: window.devicePixelRatio21 }));22 console.log(`Dimension: ${JSON.stringify(dimensions)}`);23 24 // Grab a screenshot25 console.log('Saving screenshot...');26 const screenshotBuffer = await page.screenshot({ fullPage: true });27 await Apify.setValue('screenshot.png', screenshotBuffer, { contentType: 'image/png' });28 29 console.log('Saving PDF snapshot...');30 const pdfBuffer = await page.pdf({ format: 'A4'});31 await Apify.setValue('page.pdf', pdfBuffer, { contentType: 'application/pdf' });32 33 console.log('Closing Puppeteer...');34 await browser.close();35 36 console.log('Done.');37 console.log('You can check the output in the key-value on the following URLs:');38 const storeId = process.env.APIFY_DEFAULT_KEY_VALUE_STORE_ID;39 console.log(`- https://api.apify.com/v2/key-value-stores/${storeId}/records/screenshot.png`);40 // NOTE: Adding disableRedirect=1 param, because for some reason Chrome doesn't allow pasting URLs to PDF41 // that redirect into the browser address bar (yeah, wtf...)42 console.log(`- https://api.apify.com/v2/key-value-stores/${storeId}/records/page.pdf?disableRedirect=1`);43});
package.json
{ "name": "apify-project", "version": "0.0.1", "description": "", "author": "It's not you it's me", "license": "ISC", "dependencies": { "apify": "1.3.4", "puppeteer": "10.1.0" }, "scripts": { "start": "node main.js" }}