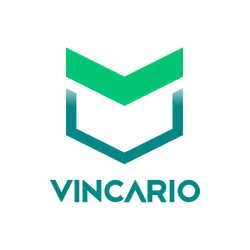
Vincario VIN Decoder API Services
Try for free
No credit card required
Go to Store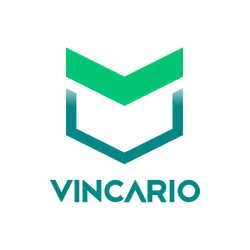
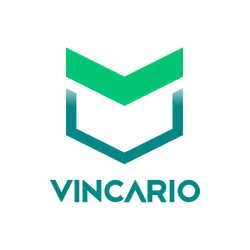
Vincario VIN Decoder API Services
vincario/vincario-vin-decoder-api-services
Try for free
No credit card required
Powerful Vincario plugin to extract data from API responses. Download Vincario data as a JSON to enrich your own applications with a powerful vehicle data partner.
.actor/Dockerfile
1# Specify the base Docker image. You can read more about
2# the available images at https://docs.apify.com/sdk/js/docs/guides/docker-images
3# You can also use any other image from Docker Hub.
4FROM apify/actor-node:18
5
6# Copy just package.json and package-lock.json
7# to speed up the build using Docker layer cache.
8COPY package*.json ./
9
10# Install NPM packages, skip optional and development dependencies to
11# keep the image small. Avoid logging too much and print the dependency
12# tree for debugging
13RUN npm --quiet set progress=false \
14 && npm install --omit=dev --omit=optional \
15 && echo "Installed NPM packages:" \
16 && (npm list --omit=dev --all || true) \
17 && echo "Node.js version:" \
18 && node --version \
19 && echo "NPM version:" \
20 && npm --version \
21 && rm -r ~/.npm
22
23# Next, copy the remaining files and directories with the source code.
24# Since we do this after NPM install, quick build will be really fast
25# for most source file changes.
26COPY . ./
27
28
29# Run the image.
30CMD npm start --silent
.actor/actor.json
1{
2 "actorSpecification": 1,
3 "name": "vincario-vin-decoder-api-services",
4 "title": "Empty JavaScript project",
5 "description": "Empty project in JavaScript.",
6 "version": "0.0",
7 "buildTag": "latest",
8 "meta": {
9 "templateId": "js-empty"
10 },
11 "dockerfile": "./Dockerfile",
12 "input": "./input_schema.json",
13 "storages": {
14 "dataset": {
15 "actorSpecification": 1,
16 "views": {
17 "overview": {
18 "title": "Overview",
19 "transformation": {
20 "fields": [
21 "VIN"
22 ]
23 },
24 "display": {
25 "component": "table",
26 "properties": {
27 "VIN": {
28 "label": "Text",
29 "format": "text"
30 }
31 }
32 }
33 }
34 }
35 }
36 }
37}
.actor/input_schema.json
1{
2 "title": "PlaywrightCrawler Template",
3 "type": "object",
4 "schemaVersion": 1,
5 "properties": {
6 "apiCall": {
7 "title": "API Endpoints",
8 "type": "string",
9 "description": "Select API Service",
10 "editor": "select",
11 "default": "info",
12 "enum": ["info", "decode", "stolen-check", "vehicle-market-value", "balance"],
13 "enumTitles": ["VIN Decode Info", "VIN Decode", " VIN Stolen Check", "Vehicle Market Value", "Get balance"]
14 },
15 "apiKey": {
16 "title": "API Key",
17 "type": "string",
18 "description": "Request API Keys at https://vindecoder.eu/api/",
19 "editor": "textfield"
20 },
21 "apiSecret": {
22 "title": "API Secret",
23 "type": "string",
24 "description": "Request API Keys at https://vindecoder.eu/api/",
25 "editor": "textfield"
26 },
27 "vin": {
28 "title": "VIN",
29 "type": "string",
30 "description": "Vehicle Identification Number",
31 "editor": "textfield"
32 }
33 },
34 "required": ["apiCall", "apiKey", "apiSecret"]
35}
.vscode/launch.json
1{
2 // Use IntelliSense to learn about possible attributes.
3 // Hover to view descriptions of existing attributes.
4 // For more information, visit: https://go.microsoft.com/fwlink/?linkid=830387
5 "version": "0.2.0",
6 "configurations": [
7 {
8 "type": "node",
9 "request": "launch",
10 "name": "Launch Program",
11 "skipFiles": [
12 "<node_internals>/**"
13 ],
14 "program": "${workspaceFolder}\\src\\main.js"
15 }
16 ]
17}
src/main.js
1// Apify SDK - toolkit for building Apify Actors (Read more at https://docs.apify.com/sdk/js/)
2import { Actor, Dataset, KeyValueStore } from 'apify';
3import {sha1} from './sha1.js'
4import {vinDecoder} from './vindecoder.js'
5// Crawlee - web scraping and browser automation library (Read more at https://crawlee.dev)
6// import { CheerioCrawler } from 'crawlee';
7
8// this is ESM project, and as such, it requires you to specify extensions in your relative imports
9// read more about this here: https://nodejs.org/docs/latest-v18.x/api/esm.html#mandatory-file-extensions
10// import { router } from './routes.js';
11
12// The init() call configures the Actor for its environment. It's recommended to start every Actor with an init()
13await Actor.init();
14
15const {apiCall, apiKey, apiSecret, vin} = await Actor.getInput();
16const result = await vinDecoder(apiKey,apiSecret,apiCall, vin);
17
18console.log(result);
19await Dataset.pushData(result);
20await KeyValueStore.setValue('OUTPUT', result);
21
22/**
23 * Actor code
24 */
25
26// Gracefully exit the Actor process. It's recommended to quit all Actors with an exit()
27await Actor.exit();
src/sha1.js
1export const sha1 = (str) => {
2
3 var _rotLeft = function (n, s) {
4 var t4 = (n << s) | (n >>> (32 - s))
5 return t4
6 }
7
8 var _cvtHex = function (val) {
9 var str = ''
10 var i
11 var v
12
13 for (i = 7; i >= 0; i--) {
14 v = (val >>> (i * 4)) & 0x0f
15 str += v.toString(16)
16 }
17 return str
18 }
19
20 var blockstart
21 var i, j
22 var W = new Array(80)
23 var H0 = 0x67452301
24 var H1 = 0xEFCDAB89
25 var H2 = 0x98BADCFE
26 var H3 = 0x10325476
27 var H4 = 0xC3D2E1F0
28 var A, B, C, D, E
29 var temp
30
31 // utf8_encode
32 str = unescape(encodeURIComponent(str))
33 var strLen = str.length
34
35 var wordArray = []
36 for (i = 0; i < strLen - 3; i += 4) {
37 j = str.charCodeAt(i) << 24 |
38 str.charCodeAt(i + 1) << 16 |
39 str.charCodeAt(i + 2) << 8 |
40 str.charCodeAt(i + 3)
41 wordArray.push(j)
42 }
43
44 switch (strLen % 4) {
45 case 0:
46 i = 0x080000000
47 break
48 case 1:
49 i = str.charCodeAt(strLen - 1) << 24 | 0x0800000
50 break
51 case 2:
52 i = str.charCodeAt(strLen - 2) << 24 | str.charCodeAt(strLen - 1) << 16 | 0x08000
53 break
54 case 3:
55 i = str.charCodeAt(strLen - 3) << 24 |
56 str.charCodeAt(strLen - 2) << 16 |
57 str.charCodeAt(strLen - 1) <<
58 8 | 0x80
59 break
60 }
61
62 wordArray.push(i)
63
64 while ((wordArray.length % 16) !== 14) {
65 wordArray.push(0)
66 }
67
68 wordArray.push(strLen >>> 29)
69 wordArray.push((strLen << 3) & 0x0ffffffff)
70
71 for (blockstart = 0; blockstart < wordArray.length; blockstart += 16) {
72 for (i = 0; i < 16; i++) {
73 W[i] = wordArray[blockstart + i]
74 }
75 for (i = 16; i <= 79; i++) {
76 W[i] = _rotLeft(W[i - 3] ^ W[i - 8] ^ W[i - 14] ^ W[i - 16], 1)
77 }
78
79 A = H0
80 B = H1
81 C = H2
82 D = H3
83 E = H4
84
85 for (i = 0; i <= 19; i++) {
86 temp = (_rotLeft(A, 5) + ((B & C) | (~B & D)) + E + W[i] + 0x5A827999) & 0x0ffffffff
87 E = D
88 D = C
89 C = _rotLeft(B, 30)
90 B = A
91 A = temp
92 }
93
94 for (i = 20; i <= 39; i++) {
95 temp = (_rotLeft(A, 5) + (B ^ C ^ D) + E + W[i] + 0x6ED9EBA1) & 0x0ffffffff
96 E = D
97 D = C
98 C = _rotLeft(B, 30)
99 B = A
100 A = temp
101 }
102
103 for (i = 40; i <= 59; i++) {
104 temp = (_rotLeft(A, 5) + ((B & C) | (B & D) | (C & D)) + E + W[i] + 0x8F1BBCDC) & 0x0ffffffff
105 E = D
106 D = C
107 C = _rotLeft(B, 30)
108 B = A
109 A = temp
110 }
111
112 for (i = 60; i <= 79; i++) {
113 temp = (_rotLeft(A, 5) + (B ^ C ^ D) + E + W[i] + 0xCA62C1D6) & 0x0ffffffff
114 E = D
115 D = C
116 C = _rotLeft(B, 30)
117 B = A
118 A = temp
119 }
120
121 H0 = (H0 + A) & 0x0ffffffff
122 H1 = (H1 + B) & 0x0ffffffff
123 H2 = (H2 + C) & 0x0ffffffff
124 H3 = (H3 + D) & 0x0ffffffff
125 H4 = (H4 + E) & 0x0ffffffff
126 }
127
128 temp = _cvtHex(H0) + _cvtHex(H1) + _cvtHex(H2) + _cvtHex(H3) + _cvtHex(H4)
129 return temp.toLowerCase()
130 }
src/vindecoder.js
1import got from 'got'
2import {sha1} from './sha1.js'
3
4export const vinDecoder = async (apiKey, secretKey, id, vin = null) => {
5
6 let apiPrefix = "https://api.vindecoder.eu/3.2";
7 let action = (id === "info") ? "decode/info" : id;
8
9 let hash = sha1((vin ? vin + '|' : '') + id + '|' + apiKey + '|' + secretKey).substring(0, 10);
10 let path = apiPrefix + '/' + apiKey + '/' + hash + '/' + action + (vin ? '/' + vin : '') + '.json';
11
12 return await got({url:path}).json();
13
14 return new Promise((resolve, reject) => {
15 https.get(path, (resp) => {
16 let data = '';
17
18 resp.on('data', (chunk) => {
19 data += chunk;
20 });
21
22 resp.on('end', () => {
23 resolve(data)
24 });
25
26 }).on("error", (err) => {
27 reject(err);
28 });
29 });
30}
.dockerignore
1# configurations
2.idea
3
4# crawlee and apify storage folders
5apify_storage
6crawlee_storage
7storage
8
9# installed files
10node_modules
11
12# git folder
13.git
.editorconfig
1root = true
2
3[*]
4indent_style = space
5indent_size = 4
6charset = utf-8
7trim_trailing_whitespace = true
8insert_final_newline = true
9end_of_line = lf
.eslintrc
1{
2 "extends": "@apify",
3 "root": true
4}
.gitignore
1# This file tells Git which files shouldn't be added to source control
2
3.DS_Store
4.idea
5node_modules
6storage
7
8# Added by Apify CLI
9.venv
package.json
1{
2 "name": "vindecoder",
3 "version": "0.0.1",
4 "type": "module",
5 "description": "This is a boilerplate of an Apify Actor.",
6 "engines": {
7 "node": ">=18.0.0"
8 },
9 "dependencies": {
10 "apify": "^3.1.10",
11 "crawlee": "^3.5.4",
12 "got": "^13.0.0"
13 },
14 "scripts": {
15 "start": "node ./src/main.js",
16 "lint": "./node_modules/.bin/eslint ./src --ext .js,.jsx",
17 "lint:fix": "./node_modules/.bin/eslint ./src --ext .js,.jsx --fix",
18 "test": "echo \"Error: oops, the actor has no tests yet, sad!\" && exit 1"
19 },
20 "author": "It's not you it's me",
21 "license": "ISC"
22}
Developer
Maintained by Community
Actor Metrics
3 monthly users
-
0 No stars yet
75% runs succeeded
Created in Sep 2023
Modified a year ago
Categories