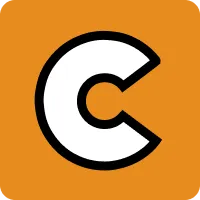
Cheerio Scraper
Pricing
Pay per usage
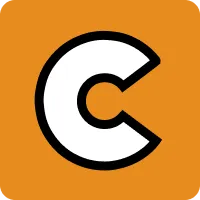
Cheerio Scraper
Crawls websites using raw HTTP requests, parses the HTML with the Cheerio library, and extracts data from the pages using a Node.js code. Supports both recursive crawling and lists of URLs. This actor is a high-performance alternative to apify/web-scraper for websites that do not require JavaScript.
4.7 (10)
Pricing
Pay per usage
164
Total users
8.6K
Monthly users
912
Runs succeeded
>99%
Issues response
11 days
Last modified
a month ago
You can access the Cheerio Scraper programmatically from your own applications by using the Apify API. You can also choose the language preference from below. To use the Apify API, you’ll need an Apify account and your API token, found in Integrations settings in Apify Console.
# Set API token$API_TOKEN=<YOUR_API_TOKEN>
# Prepare Actor input$cat > input.json << 'EOF'<{< "startUrls": [< {< "url": "https://crawlee.dev/js"< }< ],< "respectRobotsTxtFile": true,< "globs": [< {< "glob": "https://crawlee.dev/js/*/*"< }< ],< "pseudoUrls": [],< "excludes": [< {< "glob": "/**/*.{png,jpg,jpeg,pdf}"< }< ],< "linkSelector": "a[href]",< "pageFunction": "async function pageFunction(context) {\n const { $, request, log } = context;\n\n // The \"$\" property contains the Cheerio object which is useful\n // for querying DOM elements and extracting data from them.\n const pageTitle = $('title').first().text();\n\n // The \"request\" property contains various information about the web page loaded. \n const url = request.url;\n \n // Use \"log\" object to print information to Actor log.\n log.info('Page scraped', { url, pageTitle });\n\n // Return an object with the data extracted from the page.\n // It will be stored to the resulting dataset.\n return {\n url,\n pageTitle\n };\n}",< "proxyConfiguration": {< "useApifyProxy": true< },< "initialCookies": [],< "additionalMimeTypes": [],< "preNavigationHooks": "// We need to return array of (possibly async) functions here.\n// The functions accept two arguments: the \"crawlingContext\" object\n// and \"requestAsBrowserOptions\" which are passed to the `requestAsBrowser()`\n// function the crawler calls to navigate..\n[\n async (crawlingContext, requestAsBrowserOptions) => {\n // ...\n }\n]",< "postNavigationHooks": "// We need to return array of (possibly async) functions here.\n// The functions accept a single argument: the \"crawlingContext\" object.\n[\n async (crawlingContext) => {\n // ...\n },\n]",< "customData": {}<}<EOF
# Run the Actor using an HTTP API# See the full API reference at https://docs.apify.com/api/v2$curl "https://api.apify.com/v2/acts/apify~cheerio-scraper/runs?token=$API_TOKEN" \< -X POST \< -d @input.json \< -H 'Content-Type: application/json'
Cheerio Scraper - HTML scraping tool API
Below, you can find a list of relevant HTTP API endpoints for calling the Cheerio Scraper Actor. For this, you’ll need an Apify account. Replace <YOUR_API_TOKEN> in the URLs with your Apify API token, which you can find under Integrations in Apify Console. For details, see the API reference.
Run Actor
https://api.apify.com/v2/acts/apify~cheerio-scraper/runs?token=<YOUR_API_TOKEN>
Note: By adding the method=POST
query parameter, this API endpoint can be called using a GET request and thus used in third-party webhooks. Please refer to our Run Actor API documentation.
Run Actor synchronously and get dataset items
https://api.apify.com/v2/acts/apify~cheerio-scraper/run-sync-get-dataset-items?token=<YOUR_API_TOKEN>
Note: This endpoint supports both POST and GET request methods. However, only the POST method allows you to pass input data. For more information, please refer to our Run Actor synchronously and get dataset items API documentation.
Get Actor
https://api.apify.com/v2/acts/apify~cheerio-scraper?token=<YOUR_API_TOKEN>
For more information, please refer to our Get Actor API documentation.
Actors can be used to scrape web pages, extract data, or automate browser tasks. Use the Cheerio Scraper API programmatically via the Apify API.
You can choose from:
You can start Cheerio Scraper with the Apify API by sending an HTTP POST request to the Run Actorendpoint. An Actor’s input and its content type can be passed as a payload of the POST request, and additional options can be specified using URL query parameters. The Cheerio Scraper is identified within the API by its ID, which is the creator’s username and the name of the Actor.
When the Cheerio Scraper run finishes you can list the data from its default dataset(storage) via the API or you can preview the data directly on Apify Console.