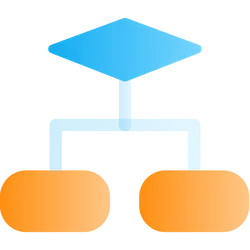
JavaScript Code to Flowchart
Pricing
Pay per usage
Go to Store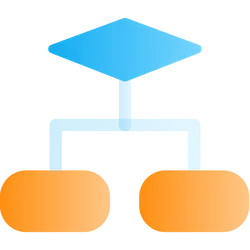
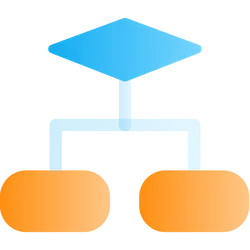
JavaScript Code to Flowchart
Use this to convert JavaScript code to a flowchart. The actor uses https://www.npmjs.com/package/js2flowchart npm package to convert the code to a flowchart. The output is an SVG file.
0.0 (0)
Pricing
Pay per usage
4
Monthly users
12
Runs succeeded
87%
Last modified
2 years ago
Dockerfile
1# First, specify the base Docker image. You can read more about
2# the available images at https://sdk.apify.com/docs/guides/docker-images
3# You can also use any other image from Docker Hub.
4FROM apify/actor-node:16
5
6# Second, copy just package.json and package-lock.json since those are the only
7# files that affect "npm install" in the next step, to speed up the build.
8COPY package*.json ./
9
10# Install NPM packages, skip optional and development dependencies to
11# keep the image small. Avoid logging too much and print the dependency
12# tree for debugging
13RUN npm --quiet set progress=false \
14 && npm install --only=prod --no-optional \
15 && echo "Installed NPM packages:" \
16 && (npm list || true) \
17 && echo "Node.js version:" \
18 && node --version \
19 && echo "NPM version:" \
20 && npm --version
21
22# Next, copy the remaining files and directories with the source code.
23# Since we do this after NPM install, quick build will be really fast
24# for most source file changes.
25COPY . ./
26
27# Optionally, specify how to launch the source code of your actor.
28# By default, Apify's base Docker images define the CMD instruction
29# that runs the Node.js source code using the command specified
30# in the "scripts.start" section of the package.json file.
31# In short, the instruction looks something like this:
32#
33# CMD npm start
INPUT_SCHEMA.json
1{
2 "title": "Google Maps Scraper",
3 "description": "Provide either a list of search keywords or search/place URLs. It is better to set location in the Geolocation settings below rather than add into the search terms. You will get more results and they will be accurate.",
4 "type": "object",
5 "schemaVersion": 1,
6 "properties": {
7 "code":{
8 "title": "JavaScript Code",
9 "type": "string",
10 "editor": "javascript",
11 "description": "JS code",
12 "prefill": "// The function accepts a single argument: the \"context\" object.\n// For a complete list of its properties and functions,\n// see https://apify.com/apify/web-scraper#page-function \nasync function pageFunction(context) {\n // This statement works as a breakpoint when you're trying to debug your code. Works only with Run mode: DEVELOPMENT!\n // debugger; \n\n // jQuery is handy for finding DOM elements and extracting data from them.\n // To use it, make sure to enable the \"Inject jQuery\" option.\n const $ = context.jQuery;\n const pageTitle = $('title').first().text();\n\n // Print some information to actor log\n context.log.info(`URL: ${context.request.url}, TITLE: ${pageTitle}`);\n\n // Manually add a new page to the queue for scraping.\n context.enqueueRequest({ url: 'http://www.example.com' });\n\n // Return an object with the data extracted from the page.\n // It will be stored to the resulting dataset.\n return {\n url: context.request.url,\n pageTitle\n };\n}"
13 }
14 }
15}
main.js
1const Apify = require('apify');
2const js2flowchart = require('js2flowchart');
3
4Apify.main(async () => {
5 // Get input of your act
6 const { code } = await Apify.getValue('INPUT');
7
8
9 console.log('Generating SVG image...')
10 const svg = js2flowchart.convertCodeToSvg(code);
11
12 console.log('Saving svg image...');
13 await Apify.setValue('OUTPUT', svg, { contentType: 'image/svg+xml' });
14 console.log(`https://api.apify.com/v2/key-value-stores/${Apify.getEnv().defaultKeyValueStoreId}/records/OUTPUT`);
15 console.log('Done');
16});
package.json
1{
2 "name": "my-actor",
3 "version": "0.0.1",
4 "dependencies": {
5 "apify": "^2.2.0",
6 "js2flowchart": "1.3.3"
7 },
8 "scripts": {
9 "start": "node main.js"
10 },
11 "author": "Me!"
12}
Pricing
Pricing model
Pay per usageThis Actor is paid per platform usage. The Actor is free to use, and you only pay for the Apify platform usage.