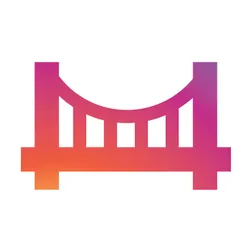
Instagram Posts to Comments Bridge
Deprecated
Pricing
Pay per usage
Go to Store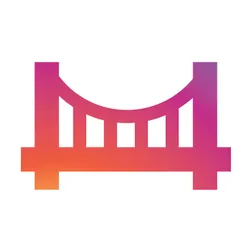
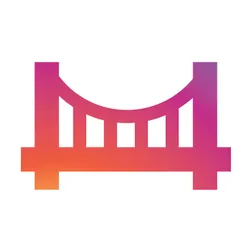
Instagram Posts to Comments Bridge
Deprecated
This simple actor helps you to get comments from Instagram user profiles. It acts as a bridge between one run scraping posts and second one scraping comments from these posts
0.0 (0)
Pricing
Pay per usage
2
Total users
64
Monthly users
5
Last modified
3 years ago
Dockerfile
# First, specify the base Docker image. You can read more about# the available images at https://sdk.apify.com/docs/guides/docker-images# You can also use any other image from Docker Hub.FROM apify/actor-node:16
# Second, copy just package.json and package-lock.json since those are the only# files that affect "npm install" in the next step, to speed up the build.COPY package*.json ./
# Install NPM packages, skip optional and development dependencies to# keep the image small. Avoid logging too much and print the dependency# tree for debuggingRUN npm --quiet set progress=false \ && npm install --only=prod --no-optional \ && echo "Installed NPM packages:" \ && (npm list || true) \ && echo "Node.js version:" \ && node --version \ && echo "NPM version:" \ && npm --version
# Next, copy the remaining files and directories with the source code.# Since we do this after NPM install, quick build will be really fast# for most source file changes.COPY . ./
# Optionally, specify how to launch the source code of your actor.# By default, Apify's base Docker images define the CMD instruction# that runs the Node.js source code using the command specified# in the "scripts.start" section of the package.json file.# In short, the instruction looks something like this:## CMD npm start
main.js
1const Apify = require('apify');2
3const { log } = Apify.utils;4
5Apify.main(async () => {6 let {7 datasetId,8 resource,9 taskIdOrName10 } = await Apify.getInput();11 if (!datasetId && resource) {12 datasetId = resource.defaultDatasetId;13 }14
15 log.info(`Loading dataset: ${datasetId}`)16
17 const dataset = await Apify.openDataset(datasetId);18 const { items } = await dataset.getData({19 clean: true,20 fields: ['url']21 });22
23 log.info(`Loaded ${items.length} URLs from the dataset`);24
25 const directUrls = items.map((item) => item.url);26
27 log.info(`Calling actor to scrape posts`);28
29 let run;30 // User can provide task to call, otherwise we call actor directly31 if (taskIdOrName) {32 run = await Apify.callTask(33 taskIdOrName,34 { directUrls, resultsType: 'comments'},35 { waitSecs: 0 }36 )37 } else {38 const actorInput = {39 directUrls,40 resultsType: 'comments',41 "proxy": {42 "useApifyProxy": true,43 "apifyProxyGroups": [44 "RESIDENTIAL"45 ]46 },47 "resultsLimit": 1000,48 }49 run = await Apify.call(50 'jaroslavhejlek/instagram-scraper',51 actorInput,52 { waitSecs: 0 }53 )54 }55
56 log.info(`Started run to scrape posts with ID: ${run.id}`);57 log.info(`You can view the run here:`);58 log.info(`https://my.apify.com/actors/shu8hvrXbJbY3Eb9W#/runs/${run.id}`)59
60});
package.json
{ "name": "my-actor", "version": "0.0.1", "dependencies": { "apify": "^1.2.1" }, "scripts": { "start": "node main.js" }, "author": "Me!"}