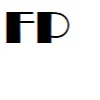
FParse
Try for free
No credit card required
Go to Store
This Actor is under maintenance.
This Actor may be unreliable while under maintenance. Would you like to try a similar Actor instead?
See alternative Actors.actor/Dockerfile
1# Specify the base Docker image. You can read more about
2# the available images at https://sdk.apify.com/docs/guides/docker-images
3# You can also use any other image from Docker Hub.
4FROM apify/actor-node:16
5
6# Copy just package.json and package-lock.json
7# to speed up the build using Docker layer cache.
8COPY package*.json ./
9
10# Install NPM packages, skip optional and development dependencies to
11# keep the image small. Avoid logging too much and print the dependency
12# tree for debugging
13RUN npm --quiet set progress=false \
14 && npm install --omit=dev --omit=optional \
15 && echo "Installed NPM packages:" \
16 && (npm list --omit=dev --all || true) \
17 && echo "Node.js version:" \
18 && node --version \
19 && echo "NPM version:" \
20 && npm --version \
21 && rm -r ~/.npm
22
23# Next, copy the remaining files and directories with the source code.
24# Since we do this after NPM install, quick build will be really fast
25# for most source file changes.
26COPY . ./
27
28
29# Run the image.
30CMD npm start --silent
.actor/INPUT_SCHEMA.json
1{
2 "title": "Input schema for the empty project actor.",
3 "type": "object",
4 "schemaVersion": 1,
5 "properties": {
6 "test": {
7 "title": "Test",
8 "type": "string",
9 "description": "This is test input field description.",
10 "editor": "textfield"
11 }
12 },
13 "required": []
14}
.actor/README.md
1# Empty project
2
3This template is useful when you're already familiar with the [Apify SDK](https://sdk.apify.com) and [Crawlee](https://crawlee.dev) and want to start with a clean slate. It does not include `puppeteer` or `playwright` so install them manually and update the Dockerfile if you need them.
4
5> We decided to split Apify SDK into two libraries, [Crawlee](https://crawlee.dev) and [Apify SDK v3](https://sdk.apify.com). Crawlee will retain all the crawling and scraping-related tools and will always strive to be the best web scraping library for its community. At the same time, Apify SDK will continue to exist, but keep only the Apify-specific features related to building actors on the Apify platform. Read the [upgrading guide](https://sdk.apify.com/docs/upgrading/upgrading-to-v3) to learn about the changes.
6
7If you're looking for examples or want to learn more visit:
8
9- [Crawlee + Apify Platform guide](https://crawlee.dev/docs/guides/apify-platform)
10- [Crawlee Tutorial](https://crawlee.dev/docs/introduction)
11- [Crawlee Examples](https://crawlee.dev/docs/examples)
12
13## Documentation reference
14
15- [Crawlee](https://crawlee.dev)
16- [Apify SDK v3](https://sdk.apify.com)
17- [Apify Actor documentation](https://docs.apify.com/actor)
18- [Apify CLI](https://docs.apify.com/cli)
19
20## Writing a README
21
22See our tutorial on [writing READMEs for your actors](https://help.apify.com/en/articles/2912548-how-to-write-great-readme-for-your-actors) if you need more inspiration.
.dockerignore
1# configurations
2.idea
3
4# crawlee and apify storage folders
5apify_storage
6crawlee_storage
7storage
8
9# installed files
10node_modules
11
12# git folder
13.git
.editorconfig
1root = true
2
3[*]
4indent_style = space
5indent_size = 4
6charset = utf-8
7trim_trailing_whitespace = true
8insert_final_newline = true
9end_of_line = lf
.eslintrc
1{
2 "extends": "@apify",
3 "root": true
4}
.gitignore
1# This file tells Git which files shouldn't be added to source control
2
3.DS_Store
4.idea
5node_modules
6storage
main.js
1// This is the main Node.js source code file of your actor.
2// An actor is a program that takes an input and produces an output.
3
4// For more information, see https://sdk.apify.com
5import {Actor} from 'apify';
6import fetch from 'node-fetch';
7import fs from 'fs';
8//import unzipper from 'unzipper';
9
10
11
12// For more information, see https://crawlee.dev
13// import { CheerioCrawler } from 'crawlee';
14
15// Initialize the Apify SDK
16await Actor.init();
17
18// Get input of the actor (here only for demonstration purposes).
19const input = await Actor.getInput();
20console.log('Input:',input);
21
22/**
23 * Actor code
24 */
25
26// await fetch('https://api.apify.com/v2/actor-runs/lbyRFnTZNqimaEFjA/abort?token=apify_api_eRPmlLty4NNJHgs7kC0nCON6fTcsiv0PEIqV',{
27// method:'POST',
28// headers:{
29// 'Content-type':'application/json'
30// }
31// });
32
33const result= await fetch('https://api.apify.com/v2/datasets/0alBN9Q4C8XvzEcOv/items?clean=true&format=json')
34.then(r=>r.json());
35console.log('debug',result)
36await fetch('http://62.216.33.167:21005/api/testCol',{
37 method:'POST',
38 headers:{
39 'Content-type':'application/json'
40 },
41 body: JSON.stringify({data:result})
42}).then(()=>{console.log('Done')});
43//const unresult= unzipper.Extract(result);
44console.log('result is',result.length);
45// Exit successfully
46await Actor.exit();
package.json
1{
2 "name": "project-empty",
3 "version": "0.0.1",
4 "type": "module",
5 "description": "This is a boilerplate of an Apify actor.",
6 "engines": {
7 "node": ">=16.0.0"
8 },
9 "dependencies": {
10 "apify": "^3.0.0",
11 "crawlee": "^3.0.0",
12 "node-fetch":"3.3.0",
13 "unzipper":"0.10.11"
14 },
15 "devDependencies": {
16 "@apify/eslint-config": "^0.3.1",
17 "eslint": "^8.20.0"
18 },
19 "scripts": {
20 "start": "node main.js",
21 "lint": "./node_modules/.bin/eslint ./src --ext .js,.jsx",
22 "lint:fix": "./node_modules/.bin/eslint ./src --ext .js,.jsx --fix",
23 "test": "echo \"Error: oops, the actor has no tests yet, sad!\" && exit 1"
24 },
25 "author": "It's not you it's me",
26 "license": "ISC"
27}
Developer
Maintained by Community
Actor Metrics
1 monthly user
-
1 star
0% runs succeeded
Created in Feb 2023
Modified 2 years ago
Categories