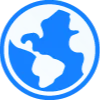
Web Scraper
Pricing
Pay per usage
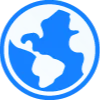
Web Scraper
Crawls arbitrary websites using a web browser and extracts structured data from web pages using a provided JavaScript function. The Actor supports both recursive crawling and lists of URLs, and automatically manages concurrency for maximum performance.
4.5 (23)
Pricing
Pay per usage
916
Total users
90K
Monthly users
5K
Runs succeeded
>99%
Issues response
7.8 days
Last modified
2 months ago
You can access the Web Scraper programmatically from your own applications by using the Apify API. You can also choose the language preference from below. To use the Apify API, you’ll need an Apify account and your API token, found in Integrations settings in Apify Console.
# Set API token$API_TOKEN=<YOUR_API_TOKEN>
# Prepare Actor input$cat > input.json << 'EOF'<{< "runMode": "DEVELOPMENT",< "startUrls": [< {< "url": "https://crawlee.dev/js"< }< ],< "respectRobotsTxtFile": true,< "linkSelector": "a[href]",< "globs": [< {< "glob": "https://crawlee.dev/js/*/*"< }< ],< "pseudoUrls": [],< "excludes": [< {< "glob": "/**/*.{png,jpg,jpeg,pdf}"< }< ],< "pageFunction": "// The function accepts a single argument: the \"context\" object.\n// For a complete list of its properties and functions,\n// see https://apify.com/apify/web-scraper#page-function \nasync function pageFunction(context) {\n // This statement works as a breakpoint when you're trying to debug your code. Works only with Run mode: DEVELOPMENT!\n // debugger; \n\n // jQuery is handy for finding DOM elements and extracting data from them.\n // To use it, make sure to enable the \"Inject jQuery\" option.\n const $ = context.jQuery;\n const pageTitle = $('title').first().text();\n const h1 = $('h1').first().text();\n const first_h2 = $('h2').first().text();\n const random_text_from_the_page = $('p').first().text();\n\n\n // Print some information to Actor log\n context.log.info(`URL: ${context.request.url}, TITLE: ${pageTitle}`);\n\n // Manually add a new page to the queue for scraping.\n await context.enqueueRequest({ url: 'http://www.example.com' });\n\n // Return an object with the data extracted from the page.\n // It will be stored to the resulting dataset.\n return {\n url: context.request.url,\n pageTitle,\n h1,\n first_h2,\n random_text_from_the_page\n };\n}",< "proxyConfiguration": {< "useApifyProxy": true< },< "initialCookies": [],< "waitUntil": [< "networkidle2"< ],< "preNavigationHooks": "// We need to return array of (possibly async) functions here.\n// The functions accept two arguments: the \"crawlingContext\" object\n// and \"gotoOptions\".\n[\n async (crawlingContext, gotoOptions) => {\n // ...\n },\n]\n",< "postNavigationHooks": "// We need to return array of (possibly async) functions here.\n// The functions accept a single argument: the \"crawlingContext\" object.\n[\n async (crawlingContext) => {\n // ...\n },\n]",< "breakpointLocation": "NONE",< "customData": {}<}<EOF
# Run the Actor using an HTTP API# See the full API reference at https://docs.apify.com/api/v2$curl "https://api.apify.com/v2/acts/apify~web-scraper/runs?token=$API_TOKEN" \< -X POST \< -d @input.json \< -H 'Content-Type: application/json'
Web Scraper API
Below, you can find a list of relevant HTTP API endpoints for calling the Web Scraper Actor. For this, you’ll need an Apify account. Replace <YOUR_API_TOKEN> in the URLs with your Apify API token, which you can find under Integrations in Apify Console. For details, see the API reference.
Run Actor
https://api.apify.com/v2/acts/apify~web-scraper/runs?token=<YOUR_API_TOKEN>
Note: By adding the method=POST
query parameter, this API endpoint can be called using a GET request and thus used in third-party webhooks. Please refer to our Run Actor API documentation.
Run Actor synchronously and get dataset items
https://api.apify.com/v2/acts/apify~web-scraper/run-sync-get-dataset-items?token=<YOUR_API_TOKEN>
Note: This endpoint supports both POST and GET request methods. However, only the POST method allows you to pass input data. For more information, please refer to our Run Actor synchronously and get dataset items API documentation.
Get Actor
https://api.apify.com/v2/acts/apify~web-scraper?token=<YOUR_API_TOKEN>
For more information, please refer to our Get Actor API documentation.
Actors can be used to scrape web pages, extract data, or automate browser tasks. Use the Web Scraper API programmatically via the Apify API.
You can choose from:
You can start Web Scraper with the Apify API by sending an HTTP POST request to the Run Actorendpoint. An Actor’s input and its content type can be passed as a payload of the POST request, and additional options can be specified using URL query parameters. The Web Scraper is identified within the API by its ID, which is the creator’s username and the name of the Actor.
When the Web Scraper run finishes you can list the data from its default dataset(storage) via the API or you can preview the data directly on Apify Console.