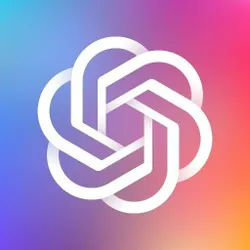
DALL-E 2 Image Generation
Pricing
Pay per usage
Go to Store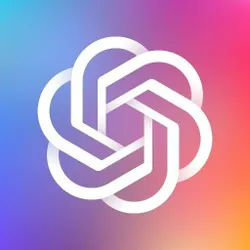
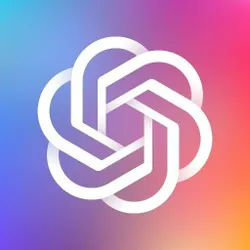
DALL-E 2 Image Generation
This actor enables you to generate images using OpenAI's DALL-E 2.
0.0 (0)
Pricing
Pay per usage
2
Total users
71
Monthly users
3
Runs succeeded
>99%
Last modified
2 years ago
.actor/Dockerfile
# First, specify the base Docker image.# You can see the Docker images from Apify at https://hub.docker.com/r/apify/.# You can also use any other image from Docker Hub.FROM apify/actor-python:3.11
# Second, copy just requirements.txt into the actor image,# since it should be the only file that affects the dependency install in the next step,# in order to speed up the buildCOPY requirements.txt ./
# Install the packages specified in requirements.txt,# Print the installed Python version, pip version# and all installed packages with their versions for debuggingRUN echo "Python version:" \ && python --version \ && echo "Pip version:" \ && pip --version \ && echo "Installing dependencies:" \ && pip install -r requirements.txt \ && echo "All installed Python packages:" \ && pip freeze
# Next, copy the remaining files and directories with the source code.# Since we do this after installing the dependencies, quick build will be really fast# for most source file changes.COPY . ./
# Specify how to launch the source code of your actor.# By default, the "python3 -m src" command is runCMD ["python3", "-m", "src"]
.actor/actor.json
{ "actorSpecification": 1, "name": "dalle-2-image-generation", "title": "DALL-E 2 image generation", "description": "Generates images using OpenAI's DALL-E 2.", "version": "0.0", "meta": { "templateId": "python-start" }, "input": "./input_schema.json", "dockerfile": "./Dockerfile"}
.actor/input_schema.json
{ "title": "DALL-E 2 image generation", "type": "object", "schemaVersion": 1, "properties": { "openai_api_key": { "title": "OpenAI API key", "type": "string", "description": "An API key from https://platform.openai.com/account/api-keys", "editor": "textfield", "isSecret": true }, "prompt": { "title": "Prompt", "type": "string", "description": "The text prompt used to generate the image(s).", "editor": "textfield" }, "count": { "title": "Count", "type": "integer", "description": "The number of images to generate.", "editor": "number", "default": 1 }, "width": { "title": "Width", "type": "integer", "description": "Width of the images.", "editor": "number", "default": 512 }, "height": { "title": "Height", "type": "integer", "description": "Height of the images.", "editor": "number", "default": 512 } }, "required": ["openai_api_key", "prompt", "count", "width", "height"]}
src/__init__.py
1
src/__main__.py
1import asyncio2import logging3
4from apify.log import ActorLogFormatter5
6from .main import main7
8handler = logging.StreamHandler()9handler.setFormatter(ActorLogFormatter())10
11apify_client_logger = logging.getLogger('apify_client')12apify_client_logger.setLevel(logging.INFO)13apify_client_logger.addHandler(handler)14
15apify_logger = logging.getLogger('apify')16apify_logger.setLevel(logging.DEBUG)17apify_logger.addHandler(handler)18
19asyncio.run(main())
src/main.py
1import openai, requests2from apify import Actor3
4
5async def main():6 async with Actor:7 actor_input = await Actor.get_input() or {}8
9 openai_api_key = actor_input.get('openai_api_key')10 prompt = actor_input.get('prompt')11 count = actor_input.get('count')12 width = actor_input.get('width')13 height = actor_input.get('height')14
15 try:16 response = openai.Image.create(17 openai_api_key,18 prompt = prompt,19 n = count,20 size = f"{width}x{height}",21 )22 image_url_data = response['data']23 i = 024 for image_url_obj in image_url_data:25 url = image_url_obj['url']26 image = requests.get(url)27 await Actor.set_value(f'image_{i}.png', image.content)28 i += 129
30 except openai.error.OpenAIError as e:31 print(e.http_status)32 print(e.error)
.dockerignore
# configurations.idea
# crawlee and apify storage foldersapify_storagecrawlee_storagestorage
# installed files.venv
# git folder.git
.editorconfig
root = true
[*]indent_style = spaceindent_size = 4charset = utf-8trim_trailing_whitespace = trueinsert_final_newline = trueend_of_line = lf
.gitignore
# This file tells Git which files shouldn't be added to source control
.idea.DS_Store
apify_storagestorage
.venv/.env/__pypackages__dist/build/*.egg-info/*.egg
__pycache__
.mypy_cache.dmypy.jsondmypy.json.pytest_cache
.scrapy*.log
storage
requirements.txt
1# Add your dependencies here.2# See https://pip.pypa.io/en/latest/reference/requirements-file-format/3# for how to format them4apify ~= 1.0.05openai ~= 0.27.2