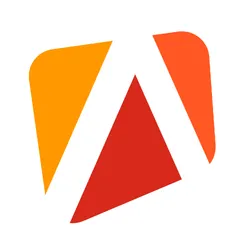
Apify Unofficial SDK
Try for free
No credit card required
View all Actors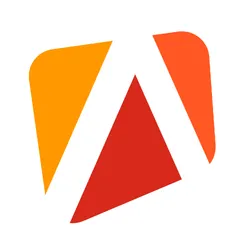
This Actor is under maintenance.
This Actor may be unreliable while under maintenance. Would you like to try a similar Actor instead?
See alternative Actors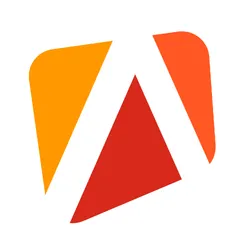
Apify Unofficial SDK
jupri/apify-unofficial-sdk
Try for free
No credit card required
Apify Unofficial SDK in Other Languanges
.actor/Dockerfile
1FROM golang:1.20.10-alpine
2
3WORKDIR /app
4
5# pre-copy/cache go.mod for pre-downloading dependencies and only redownloading them in subsequent builds if they change
6
7#COPY go.mod go.sum ./
8#RUN go mod download && go mod verify
9
10COPY . .
11
12RUN cd src \
13&& go mod init example \
14&& go mod tidy \
15&& go build -o /usr/local/bin/app .
16
17# && go mod edit -replace localhost/apify=../apify \
18
19
20CMD ["app"]
.actor/actor.json
1{
2 "actorSpecification": 1,
3 "name": "my-actor-4",
4 "title": "Empty Python project",
5 "description": "Empty project in Python.",
6 "version": "0.0",
7 "buildTag": "latest",
8 "meta": {
9 "templateId": "python-empty"
10 },
11 "dockerfile": "./Dockerfile"
12}
src/apify/actor.go
1package apify
2import (
3 "fmt"
4 "net/http"
5)
6
7////////////////////////////////////////////////////////////
8
9type clientHttp struct {
10 token string
11 base_url string
12}
13
14func (c * clientHttp) url (path string) string {
15 return c.base_url + "/" + path
16}
17
18func (c * clientHttp) request (method, path string) string {
19 cli := & http.Client {
20 //CheckRedirect: redirectPolicyFunc,
21 }
22
23 url := c.url(path)
24 req, err := http.NewRequest(method, url, nil)
25
26 if c.token != "" {
27 req.Header.Add("Authorization", c.token)
28 }
29
30 res, err := cli.Do(req)
31
32 fmt.Println(res, err)
33
34 return ""
35}
36
37////////////////////////////////////////////////////////////
38
39/**
40type storeBase struct {
41}
42**/
43
44type storeHttp struct {
45 clientHttp
46}
47
48type DatasetStoreHttp struct {
49 storeHttp
50}
51
52type KeyValueStore struct {
53 storeHttp
54}
55
56func (s * KeyValueStore) GetValue (key string) string {
57 res := s.request("GET", fmt.Sprintf("records/%s", key))
58 return res
59}
60
61////////////////////////////////////////////////////////////
62type _actor struct {
63 kv_store KeyValueStore
64}
65
66func newActor() * _actor {
67 return & _actor {
68 kv_store: KeyValueStore {
69 storeHttp {
70 clientHttp {
71 token : config.TOKEN,
72 base_url : fmt.Sprintf("%s/v2/key-value-stores/%s", config.API_PUBLIC_BASE_URL, config.DEFAULT_KEY_VALUE_STORE_ID),
73 },
74 },
75 },
76 }
77}
78
79func (a * _actor) GetInput () string {
80 return a.GetValue(config.INPUT_KEY)
81}
82func (a * _actor) GetValue (key string) string {
83 return a.kv_store.GetValue(key)
84}
85
86////////////////////////////////////////////////////////////
87
88// private
89var config = newConfiguration()
90
91// public
92var Actor = newActor()
src/apify/apify.go
1package apify
2
3func Hello() string {
4 a := TOKEN
5 println( a )
6 return "Hello"
7}
src/apify/config.go
1package apify
2
3import (
4 "os"
5)
6
7type Configuration struct {
8 ACTOR_BUILD_ID string
9 ACTOR_BUILD_NUMBER string
10 ACTOR_EVENTS_WS_URL string
11 ACTOR_ID string
12 ACTOR_RUN_ID string
13 ACTOR_TASK_ID string
14 API_BASE_URL string
15 API_PUBLIC_BASE_URL string
16 CHROME_EXECUTABLE_PATH string
17 CONTAINER_PORT string
18 CONTAINER_URL string
19 DEDICATED_CPUS string
20 DEFAULT_BROWSER_PATH string
21 DEFAULT_DATASET_ID string
22 DEFAULT_KEY_VALUE_STORE_ID string
23 DEFAULT_REQUEST_QUEUE_ID string
24 DISABLE_BROWSER_SANDBOX string
25 HEADLESS string
26 INPUT_KEY string
27 INPUT_SECRETS_PRIVATE_KEY_FILE string
28 INPUT_SECRETS_PRIVATE_KEY_PASSPHRASE string
29 IS_AT_HOME string
30 MAX_USED_CPU_RATIO string
31 MEMORY_MBYTES string
32 META_ORIGIN string
33 METAMORPH_AFTER_SLEEP_MILLIS string
34 PERSIST_STATE_INTERVAL_MILLIS string
35 PERSIST_STORAGE string
36 PROXY_HOSTNAME string
37 PROXY_PASSWORD string
38 PROXY_PORT string
39 PROXY_STATUS_URL string
40 PURGE_ON_START string
41 STARTED_AT string
42 TIMEOUT_AT string
43 TOKEN string
44 USER_ID string
45 XVFB string
46 SYSTEM_INFO_INTERVAL_MILLIS string
47}
48
49func _getenv_or(key string, def string) string {
50 val := os.Getenv(key)
51 if val == "" { return def }
52 return val
53}
54func _getenv(key string) string { return _getenv_or(key, "") }
55
56func newConfiguration() * Configuration {
57 return & Configuration {
58 ACTOR_BUILD_ID : _getenv(BUILD_ID),
59 ACTOR_BUILD_NUMBER : _getenv(BUILD_NUMBER),
60 ACTOR_EVENTS_WS_URL : _getenv(EVENTS_WEBSOCKET_URL),
61 ACTOR_ID : _getenv(ID),
62 ACTOR_RUN_ID : _getenv(RUN_ID),
63 ACTOR_TASK_ID : _getenv(TASK_ID),
64 API_BASE_URL : _getenv_or(API_BASE_URL, "https://api.apify.com"),
65 API_PUBLIC_BASE_URL : _getenv_or(API_PUBLIC_BASE_URL, "https://api.apify.com"),
66 //CHROME_EXECUTABLE_PATH : _getenv(CHROME_EXECUTABLE_PATH),
67 //CONTAINER_PORT : container_port or _getenv(WEB_SERVER_PORT, 4321),
68 //CONTAINER_URL : container_url or _getenv(WEB_SERVER_URL, "http://localhost:4321"),
69 //DEDICATED_CPUS : _getenv(DEDICATED_CPUS),
70 //DEFAULT_BROWSER_PATH : _getenv(DEFAULT_BROWSER_PATH),
71 DEFAULT_DATASET_ID : _getenv_or(DEFAULT_DATASET_ID, "default"),
72 DEFAULT_KEY_VALUE_STORE_ID : _getenv_or(DEFAULT_KEY_VALUE_STORE_ID, "default"),
73 DEFAULT_REQUEST_QUEUE_ID : _getenv_or(DEFAULT_REQUEST_QUEUE_ID, "default"),
74 //DISABLE_BROWSER_SANDBOX : _getenv(DISABLE_BROWSER_SANDBOX, False),
75 //HEADLESS : _getenv(HEADLESS, True),
76 INPUT_KEY : _getenv_or(INPUT_KEY, "INPUT"),
77 //INPUT_SECRETS_PRIVATE_KEY_FILE : _getenv(INPUT_SECRETS_PRIVATE_KEY_FILE),
78 //INPUT_SECRETS_PRIVATE_KEY_PASSPHRASE : _getenv(INPUT_SECRETS_PRIVATE_KEY_PASSPHRASE),
79 IS_AT_HOME : _getenv(IS_AT_HOME),
80 //MAX_USED_CPU_RATIO : max_used_cpu_ratio or _getenv(MAX_USED_CPU_RATIO, 0.95),
81 //MEMORY_MBYTES : _getenv(MEMORY_MBYTES),
82 META_ORIGIN : _getenv(META_ORIGIN),
83 //METAMORPH_AFTER_SLEEP_MILLIS : metamorph_after_sleep_millis or _getenv(METAMORPH_AFTER_SLEEP_MILLIS, 300000) # noqa: E501
84 //PERSIST_STATE_INTERVAL_MILLIS : persist_state_interval_millis or _getenv(PERSIST_STATE_INTERVAL_MILLIS, 60000) # noqa: E501
85 //PERSIST_STORAGE : persist_storage or _getenv(PERSIST_STORAGE, True)
86 //PROXY_HOSTNAME : proxy_hostname or _getenv(PROXY_HOSTNAME, "proxy.apify.com")
87 //PROXY_PASSWORD : proxy_password or _getenv(PROXY_PASSWORD)
88 //PROXY_PORT : proxy_port or _getenv(PROXY_PORT, 8000)
89 //PROXY_STATUS_URL : proxy_status_url or _getenv(PROXY_STATUS_URL, "http://proxy.apify.com")
90 //PURGE_ON_START : purge_on_start or _getenv(PURGE_ON_START, False)
91 //STARTED_AT : _getenv(STARTED_AT)
92 //TIMEOUT_AT : _getenv(TIMEOUT_AT)
93 TOKEN : _getenv(TOKEN),
94 USER_ID : _getenv(USER_ID),
95 //XVFB : _getenv(XVFB, False)
96 //SYSTEM_INFO_INTERVAL_MILLIS : system_info_interval_millis or _getenv(SYSTEM_INFO_INTERVAL_MILLIS, 60000)
97 }
98}
src/apify/consts.go
1package apify
2
3// ActorJobStatus
4const (
5 // """Available statuses for actor jobs (runs or builds)."""
6
7 // Actor job initialized but not started yet
8 READY = "READY"
9 // Actor job in progress
10 RUNNING = "RUNNING"
11 // Actor job finished successfully
12 SUCCEEDED = "SUCCEEDED"
13 // Actor job or build failed
14 FAILED = "FAILED"
15 // Actor job currently timing out
16 TIMING_OUT = "TIMING-OUT"
17 // Actor job timed out
18 TIMED_OUT = "TIMED-OUT"
19 // Actor job currently being aborted by user
20 ABORTING = "ABORTING"
21 // Actor job aborted by user
22 ABORTED = "ABORTED"
23 /*
24 @property
25 def _is_terminal(self) -> bool:
26 """Whether this actor job status is terminal."""
27 return self in (ActorJobStatus.SUCCEEDED, ActorJobStatus.FAILED, ActorJobStatus.TIMED_OUT, ActorJobStatus.ABORTED)
28 */
29)
30
31// ActorSourceType
32const (
33 // """Available source types for actors."""
34
35 // Actor source code is comprised of multiple files
36 SOURCE_FILES = "SOURCE_FILES"
37 // Actor source code is cloned from a Git repository
38 GIT_REPO = "GIT_REPO"
39 // Actor source code is downloaded using a tarball or Zip file
40 TARBALL = "TARBALL"
41 // Actor source code is taken from a GitHub Gist
42 GITHUB_GIST = "GITHUB_GIST"
43)
44
45// ActorEventTypes
46/*
47const (
48 // """Possible values of actor event type."""
49
50 // Info about resource usage of the actor
51 SYSTEM_INFO = "systemInfo"
52 // Sent when the actor is about to migrate
53 MIGRATING = "migrating"
54 // Sent when the actor should persist its state (every minute or when migrating)
55 PERSIST_STATE = "persistState"
56 // Sent when the actor is aborting
57 ABORTING = "aborting"
58
59)
60*/
61
62// ActorEnvVars
63const (
64 // """Possible Apify-specific environment variables prefixed with "ACTOR_"."""
65
66 // TODO: document these
67
68 BUILD_ID = "ACTOR_BUILD_ID"
69 BUILD_NUMBER = "ACTOR_BUILD_NUMBER"
70 DEFAULT_DATASET_ID = "ACTOR_DEFAULT_DATASET_ID"
71 DEFAULT_KEY_VALUE_STORE_ID = "ACTOR_DEFAULT_KEY_VALUE_STORE_ID"
72 DEFAULT_REQUEST_QUEUE_ID = "ACTOR_DEFAULT_REQUEST_QUEUE_ID"
73 EVENTS_WEBSOCKET_URL = "ACTOR_EVENTS_WEBSOCKET_URL"
74 ID = "ACTOR_ID"
75 INPUT_KEY = "ACTOR_INPUT_KEY"
76 MAX_PAID_DATASET_ITEMS = "ACTOR_MAX_PAID_DATASET_ITEMS"
77 MEMORY_MBYTES = "ACTOR_MEMORY_MBYTES"
78 RUN_ID = "ACTOR_RUN_ID"
79 STARTED_AT = "ACTOR_STARTED_AT"
80 TASK_ID = "ACTOR_TASK_ID"
81 TIMEOUT_AT = "ACTOR_TIMEOUT_AT"
82 WEB_SERVER_PORT = "ACTOR_WEB_SERVER_PORT"
83 WEB_SERVER_URL = "ACTOR_WEB_SERVER_URL"
84)
85
86// ApifyEnvVars
87const (
88 // """Possible Apify-specific environment variables prefixed with "APIFY_"."""
89
90 // TODO: document these
91
92 API_BASE_URL = "APIFY_API_BASE_URL"
93 API_PUBLIC_BASE_URL = "APIFY_API_PUBLIC_BASE_URL"
94 CHROME_EXECUTABLE_PATH = "APIFY_CHROME_EXECUTABLE_PATH"
95 DEDICATED_CPUS = "APIFY_DEDICATED_CPUS"
96 DEFAULT_BROWSER_PATH = "APIFY_DEFAULT_BROWSER_PATH"
97 DISABLE_BROWSER_SANDBOX = "APIFY_DISABLE_BROWSER_SANDBOX"
98 DISABLE_OUTDATED_WARNING = "APIFY_DISABLE_OUTDATED_WARNING"
99 FACT = "APIFY_FACT"
100 HEADLESS = "APIFY_HEADLESS"
101 INPUT_SECRETS_PRIVATE_KEY_FILE = "APIFY_INPUT_SECRETS_PRIVATE_KEY_FILE"
102 INPUT_SECRETS_PRIVATE_KEY_PASSPHRASE = "APIFY_INPUT_SECRETS_PRIVATE_KEY_PASSPHRASE"
103 IS_AT_HOME = "APIFY_IS_AT_HOME"
104 LOCAL_STORAGE_DIR = "APIFY_LOCAL_STORAGE_DIR"
105 LOG_FORMAT = "APIFY_LOG_FORMAT"
106 LOG_LEVEL = "APIFY_LOG_LEVEL"
107 MAX_USED_CPU_RATIO = "APIFY_MAX_USED_CPU_RATIO"
108 META_ORIGIN = "APIFY_META_ORIGIN"
109 METAMORPH_AFTER_SLEEP_MILLIS = "APIFY_METAMORPH_AFTER_SLEEP_MILLIS"
110 PERSIST_STATE_INTERVAL_MILLIS = "APIFY_PERSIST_STATE_INTERVAL_MILLIS"
111 PERSIST_STORAGE = "APIFY_PERSIST_STORAGE"
112 PROXY_HOSTNAME = "APIFY_PROXY_HOSTNAME"
113 PROXY_PASSWORD = "APIFY_PROXY_PASSWORD"
114 PROXY_PORT = "APIFY_PROXY_PORT"
115 PROXY_STATUS_URL = "APIFY_PROXY_STATUS_URL"
116 PURGE_ON_START = "APIFY_PURGE_ON_START"
117 SDK_LATEST_VERSION = "APIFY_SDK_LATEST_VERSION"
118 SYSTEM_INFO_INTERVAL_MILLIS = "APIFY_SYSTEM_INFO_INTERVAL_MILLIS"
119 TOKEN = "APIFY_TOKEN"
120 USER_ID = "APIFY_USER_ID"
121 WORKFLOW_KEY = "APIFY_WORKFLOW_KEY"
122 XVFB = "APIFY_XVFB"
123
124 // Replaced by ActorEnvVars, kept for backward compatibility:
125 /*
126 ACTOR_BUILD_ID = "APIFY_ACTOR_BUILD_ID"
127 ACTOR_BUILD_NUMBER = "APIFY_ACTOR_BUILD_NUMBER"
128 ACTOR_EVENTS_WS_URL = "APIFY_ACTOR_EVENTS_WS_URL"
129 ACTOR_ID = "APIFY_ACTOR_ID"
130 ACTOR_RUN_ID = "APIFY_ACTOR_RUN_ID"
131 ACTOR_TASK_ID = "APIFY_ACTOR_TASK_ID"
132 CONTAINER_PORT = "APIFY_CONTAINER_PORT"
133 CONTAINER_URL = "APIFY_CONTAINER_URL"
134 DEFAULT_DATASET_ID = "APIFY_DEFAULT_DATASET_ID"
135 DEFAULT_KEY_VALUE_STORE_ID = "APIFY_DEFAULT_KEY_VALUE_STORE_ID"
136 DEFAULT_REQUEST_QUEUE_ID = "APIFY_DEFAULT_REQUEST_QUEUE_ID"
137 INPUT_KEY = "APIFY_INPUT_KEY"
138 MEMORY_MBYTES = "APIFY_MEMORY_MBYTES"
139 STARTED_AT = "APIFY_STARTED_AT"
140 TIMEOUT_AT = "APIFY_TIMEOUT_AT"
141
142 // Deprecated, kept for backward compatibility:
143 ACT_ID = "APIFY_ACT_ID"
144 ACT_RUN_ID = "APIFY_ACT_RUN_ID"
145 */
146)
147
148// ActorExitCodes
149const (
150 // """Usual actor exit codes."""
151
152 // The actor finished successfully
153 SUCCESS = 0
154
155 // The main function of the actor threw an Exception
156 ERROR_USER_FUNCTION_THREW = 91
157)
158
159// WebhookEventType
160const (
161 // """Events that can trigger a webhook."""
162
163 // The actor run was created
164 ACTOR_RUN_CREATED = "ACTOR.RUN.CREATED"
165 // The actor run has succeeded
166 ACTOR_RUN_SUCCEEDED = "ACTOR.RUN.SUCCEEDED"
167 // The actor run has failed
168 ACTOR_RUN_FAILED = "ACTOR.RUN.FAILED"
169 // The actor run has timed out
170 ACTOR_RUN_TIMED_OUT = "ACTOR.RUN.TIMED_OUT"
171 // The actor run was aborted
172 ACTOR_RUN_ABORTED = "ACTOR.RUN.ABORTED"
173 // The actor run was resurrected
174 ACTOR_RUN_RESURRECTED = "ACTOR.RUN.RESURRECTED"
175
176 // The actor build was created
177 ACTOR_BUILD_CREATED = "ACTOR.BUILD.CREATED"
178 // The actor build has succeeded
179 ACTOR_BUILD_SUCCEEDED = "ACTOR.BUILD.SUCCEEDED"
180 // The actor build has failed
181 ACTOR_BUILD_FAILED = "ACTOR.BUILD.FAILED"
182 // The actor build has timed out
183 ACTOR_BUILD_TIMED_OUT = "ACTOR.BUILD.TIMED_OUT"
184 // The actor build was aborted
185 ACTOR_BUILD_ABORTED = "ACTOR.BUILD.ABORTED"
186)
187
188// MetaOrigin
189const (
190 // """Possible origins for actor runs, i.e. how were the jobs started."""
191
192 // Job started from Developer console in Source section of actor
193 DEVELOPMENT = "DEVELOPMENT"
194 // Job started from other place on the website (either console or task detail page)
195 WEB = "WEB"
196 // Job started through API
197 API = "API"
198 // Job started through Scheduler
199 SCHEDULER = "SCHEDULER"
200 // Job started through test actor page
201 TEST = "TEST"
202 // Job started by the webhook
203 WEBHOOK = "WEBHOOK"
204 // Job started by another actor run
205 ACTOR = "ACTOR"
206)
src/__main__.py
1import asyncio
2import logging
3
4from apify.log import ActorLogFormatter
5
6from .main import main
7
8# Set up logging of messages from the Apify SDK
9handler = logging.StreamHandler()
10handler.setFormatter(ActorLogFormatter())
11
12apify_client_logger = logging.getLogger('apify_client')
13apify_client_logger.setLevel(logging.INFO)
14apify_client_logger.addHandler(handler)
15
16apify_logger = logging.getLogger('apify')
17apify_logger.setLevel(logging.DEBUG)
18apify_logger.addHandler(handler)
19
20asyncio.run(main())
src/main.go
1package main
2
3import (
4 //"os"
5 "example/apify"
6)
7
8func main() {
9
10 input := apify.Actor.GetInput()
11
12 println( input )
13}
src/main.py
1# Apify SDK - toolkit for building Apify Actors (Read more at https://docs.apify.com/sdk/python)
2from apify import Actor
3
4
5async def main():
6 async with Actor:
7 Actor.log.info('Hello from the Actor!')
8 # Write your code here
requirements.txt
1apify ~= 1.1.5
Developer
Maintained by Community
Actor metrics
- 1 monthly user
- 1 star
- 100.0% runs succeeded
- Created in Oct 2023
- Modified about 1 year ago
Categories