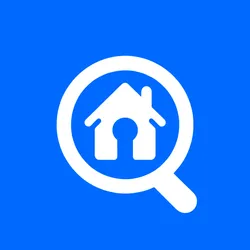
Zillow Search Scraper
Pricing
$2.00 / 1,000 results
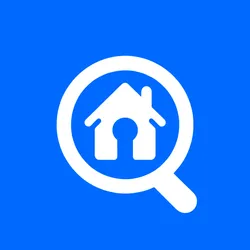
Zillow Search Scraper
Extract data about properties for sale and rent on Zillow using the Zillow API, but with no daily call limits. Scrape millions of listings and download your data as HTML, JSON, CSV, Excel, XML. Export scraped data, run the scraper via API, schedule and monitor runs, or integrate with other tools.
4.0 (9)
Pricing
$2.00 / 1,000 results
89
Total users
2.3K
Monthly users
366
Runs succeeded
97%
Issues response
25 days
Last modified
17 days ago
You can access the Zillow Search Scraper programmatically from your own applications by using the Apify API. You can also choose the language preference from below. To use the Apify API, you’ll need an Apify account and your API token, found in Integrations settings in Apify Console.
1import { ApifyClient } from 'apify-client';2
3// Initialize the ApifyClient with your Apify API token4// Replace the '<YOUR_API_TOKEN>' with your token5const client = new ApifyClient({6 token: '<YOUR_API_TOKEN>',7});8
9// Prepare Actor input10const input = {11 "searchUrls": [12 {13 "url": "https://www.zillow.com/homes/for_sale/?searchQueryState=%7B%22isMapVisible%22%3Atrue%2C%22mapBounds%22%3A%7B%22west%22%3A-124.61572460426518%2C%22east%22%3A-120.37225536598393%2C%22south%22%3A36.71199595991113%2C%22north%22%3A38.74934086729303%7D%2C%22filterState%22%3A%7B%22sort%22%3A%7B%22value%22%3A%22days%22%7D%2C%22ah%22%3A%7B%22value%22%3Atrue%7D%7D%2C%22isListVisible%22%3Atrue%2C%22customRegionId%22%3A%227d43965436X1-CRmxlqyi837u11_1fi65c%22%7D"14 }15 ]16};17
18// Run the Actor and wait for it to finish19const run = await client.actor("maxcopell/zillow-scraper").call(input);20
21// Fetch and print Actor results from the run's dataset (if any)22console.log('Results from dataset');23console.log(`💾 Check your data here: https://console.apify.com/storage/datasets/${run.defaultDatasetId}`);24const { items } = await client.dataset(run.defaultDatasetId).listItems();25items.forEach((item) => {26 console.dir(item);27});28
29// 📚 Want to learn more 📖? Go to → https://docs.apify.com/api/client/js/docs
Zillow Real Estate Scraper 🏘️ API in JavaScript
The Apify API client for JavaScript is the official library that allows you to use Zillow Search Scraper API in JavaScript or TypeScript, providing convenience functions and automatic retries on errors.
Install the apify-client
$npm install apify-client
Other API clients include: