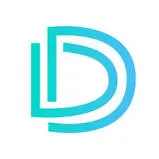
Datadome Sound Captcha
Deprecated
Pricing
Pay per usage
Go to Store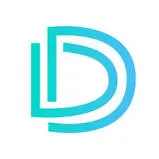
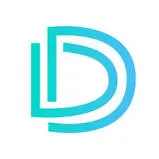
Datadome Sound Captcha
Deprecated
Solve Datadome sound captchas using AI by providing the audio file URL
0.0 (0)
Pricing
Pay per usage
1
Total users
10
Monthly users
2
Last modified
2 years ago
Datadome Sound Captcha Solver
Solve Datadome captchas using AI by providing the audio file URL.
How it works
Assuming you hit a page with Datadome slider captcha, here's how to solve it:
- Extract the audio captcha file URL (e.g.,
https://dd.prod.captcha-delivery.com/audio/2023-05-19/en/9a16b0aa574adc63ff07847678f320be.wav
) from a page with Datadome slider captcha - Send request to this API with the extracted audio URL
- Type in the 6-digit numbers returned by the API into the audio captcha number fields
- Celebrate victory!!!
Note
If the captcha is not solved on first attempt, you can easily click the reload button (#captcha__reload__button
) on the slider to re-generate a new audio URL and make the request again.
Example with puppeteer (similar approach for Playwright)
(async () => {// Importsimport { ApifyClient } from 'apify-client';// Assuming you hit a page with Datadome Slider Captcha e.g., when visiting https://www.super.com/travel/...const sleep = (ms) => new Promise((r) => setTimeout(r, ms));console.log(`Solving Captcha...`)// Get Datadome captcha iframeawait page.waitForSelector(`iframe`)const iframe = await page.frames().find((frame) => {return frame.url().includes(`https://geo.captcha-delivery.com/captcha/?`)})// Sleep for 3 seconds or any desired time to allow iframe to load its contentsawait sleep(3000)// Extract audio URL from Datadome iframeconst audio_url = await iframe.evaluate(() => {return document.querySelector(`audio[src*="https://dd.prod.captcha-delivery.com/audio"]`)?.getAttribute(`src`)})// Make API requestlet numbers;// Initialize the ApifyClient with API tokenconst client = new ApifyClient({token: '<YOUR_API_TOKEN>',});// Prepare actor inputconst input = {"audio_url": "https://dd.prod.captcha-delivery.com/audio/2023-05-19/en/3a1e6a23a02face80c0f26578049ced9.wav","proxyConfig": {"useApifyProxy": false}};try {// Run the actor and wait for it to finishconst run = await client.actor("microworlds/datadome-sound-captcha").call(input);// Fetch and print actor results from the run's dataset (if any)console.log('Results from dataset');const { items } = await client.dataset(run.defaultDatasetId).listItems();items.forEach((item) => {console.dir(item); // e.g ['3', '3', '6', '4', '3', '2']numbers = item});} catch (error) {console.error(error);}// Click the speaker icon on the captcha to toggle the audio sectionawait iframe.click(`#captcha__audio__button`)sleep(500)// Type the numbers (6-digits) into the audio captcha input fieldsfor (let [index, value] of numbers.entries()) {console.log(`Entering the value - ${value}`)await iframe.type(`#captcha__audio input.audio-captcha-inputs[data-index="${index}"]`, `${value}`, {delay: 500})}// Tab yourself three times on the back and watch Datadome give you passage π// However, if that fails, you can generate another audio url by clicking on the retry icon like so and repeat the process:await iframe.click(`#captcha__reload__button`)...})();
On this page
Share Actor: