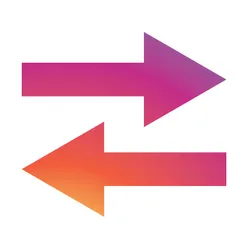
Instagram IDs to username
Deprecated
Pricing
Pay per usage
Go to Store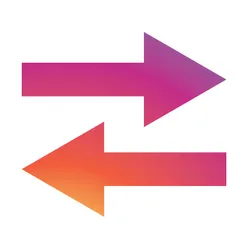
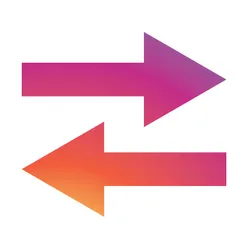
Instagram IDs to username
Deprecated
Convert Instagram ID numbers back to usernames
0.0 (0)
Pricing
Pay per usage
1
Total users
572
Monthly users
1
Runs succeeded
>99%
Last modified
2 years ago
.editorconfig
root = true
[*]indent_style = spaceindent_size = 4charset = utf-8trim_trailing_whitespace = trueinsert_final_newline = trueend_of_line = lf
.eslintrc
{ "extends": "@apify"}
.gitignore
# This file tells Git which files shouldn't be added to source control
.ideanode_modules
Dockerfile
# First, specify the base Docker image. You can read more about# the available images at https://sdk.apify.com/docs/guides/docker-images# You can also use any other image from Docker Hub.FROM apify/actor-node:16
# Second, copy just package.json and package-lock.json since it should be# the only file that affects "npm install" in the next step, to speed up the buildCOPY package*.json ./
# Install NPM packages, skip optional and development dependencies to# keep the image small. Avoid logging too much and print the dependency# tree for debuggingRUN npm --quiet set progress=false \ && npm install --only=prod --no-optional \ && echo "Installed NPM packages:" \ && (npm list --only=prod --no-optional --all || true) \ && echo "Node.js version:" \ && node --version \ && echo "NPM version:" \ && npm --version
# Next, copy the remaining files and directories with the source code.# Since we do this after NPM install, quick build will be really fast# for most source file changes.COPY . ./
# Optionally, specify how to launch the source code of your actor.# By default, Apify's base Docker images define the CMD instruction# that runs the Node.js source code using the command specified# in the "scripts.start" section of the package.json file.# In short, the instruction looks something like this:## CMD npm start
INPUT_SCHEMA.json
{ "title": "Input schema for the apify_project actor.", "type": "object", "schemaVersion": 1, "properties": { "ids": { "title": "Instagram Ids", "type": "array", "default": [], "prefill": ["297604134"], "description": "Get the usernames of given Instagram Ids", "editor": "stringList" } }, "required": []}
apify.json
{ "env": { "npm_config_loglevel": "silent" }}
main.js
1// This is the main Node.js source code file of your actor.2
3// Import Apify SDK. For more information, see https://sdk.apify.com/4const Apify = require('apify');5
6const { log } = Apify.utils;7
8const isNumber = (s) => +s == s;9const addRequest = (id, userData) => ({ url: `https://i.instagram.com/api/v1/users/${id}/info/`, userData });10
11Apify.main(async () => {12 const { ids, resource } = await Apify.getInput();13
14 const requestQueue = await Apify.openRequestQueue();15 let count = 0;16
17 if (resource?.defaultDatasetId) {18 const dataset = await Apify.openDataset(resource.defaultDatasetId);19
20 await dataset.forEach(async (item) => {21 const id = [22 item.ownerId,23 item.owner,24 ].filter(isNumber).map(String);25
26 if (id[0]) {27 await requestQueue.addRequest(addRequest(id[0], item));28 count++;29 }30 });31
32 if (resource?.defaultKeyValueStoreId) {33 const kv = await Apify.openKeyValueStore(resource?.defaultKeyValueStoreId);34 const { defaultDatasetId, actorRunId } = Apify.getEnv(); 35 36 await Promise.all([37 kv.setValue('DATASET_ID', defaultDatasetId),38 kv.setValue('RUN_ID', actorRunId),39 ]);40 }41 }42
43 for (const id of (ids ?? [])) {44 await requestQueue.addRequest(addRequest(id));45 count++;46 }47
48 const proxyConfiguration = await Apify.createProxyConfiguration({49 groups: ['RESIDENTIAL'],50 });51
52 const idCount = await requestQueue.getInfo().then(({ totalRequestCount }) => totalRequestCount || count);53
54 log.info(`Going to fetch information for ${idCount} ids`);55
56 const crawler = new Apify.CheerioCrawler({57 requestQueue,58 proxyConfiguration,59 preNavigationHooks: [async (context, requestAsBrowserOptions) => {60 requestAsBrowserOptions.headers = {61 ...requestAsBrowserOptions.headers,62 "Accept": "*/*",63 "Alt-Used": "i.instagram.com",64 "Accept-Language": "en-US,en",65 "X-IG-App-ID": "936619743392459",66 "X-ASBD-ID": "198387",67 "X-IG-WWW-Claim": "0",68 "Origin": "https://www.instagram.com",69 "Referrer": "https://www.instagram.com/",70 };71 }],72 handlePageFunction: async (context) => {73 const { request, json } = context;74
75 if (json?.status !== "ok") {76 throw new Error(`Got wrong status "${json?.status ?? json?.message ?? '-'}"`);77 }78
79 if (!json?.user) {80 throw new Error(`Missing user property from response`);81 }82
83 await Apify.pushData({ 84 ...json.user,85 url: `https://www.instagram.com/${json.user.username}`,86 ...request.userData,87 });88 }89 });90
91 await crawler.run();92});
package.json
{ "name": "project-empty", "version": "0.0.1", "description": "This is a boilerplate of an Apify actor.", "dependencies": { "apify": "^2.3.2" }, "scripts": { "start": "node main.js", "lint": "./node_modules/.bin/eslint ./src --ext .js,.jsx", "lint:fix": "./node_modules/.bin/eslint ./src --ext .js,.jsx --fix", "test": "echo \"Error: oops, the actor has no tests yet, sad!\" && exit 1" }, "author": "It's not you it's me", "license": "ISC"}