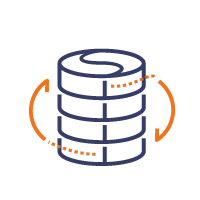
Query Dataset
Pricing
Pay per usage
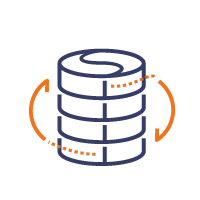
Query Dataset
DeprecatedQuery your existing datasets, map and generate a subset of your data
0.0 (0)
Pricing
Pay per usage
2
5
1
Last modified
3 years ago
Query your existing datasets, map and generate a subset of your data
Uses MongoDB-like query style, for extended documentation, check MongoDB query documentation
It uses sift module for matching, means you can use as query input.
Example
Take this dataset, for example:
[{"name": "Name 1","anotherValue": 1},{"name": "Name 2","anotherValue": 2},{"name": "","anotherValue": 3}]
You want to query only items that have a name that isn't empty, so you use the following INPUT
:
{"datasetId": "YOUR_DATASET_ID","query": {"name": { "$ne": "" }}}
$ne
means "not equal" in MongoDB, so you'll receive "Name 1" and "Name 2" items.
Now say you want to rename the "name" field to something else:
{"datasetId": "YOUR_DATASET_ID","query": {"name": { "$ne": "" }},"filterMap": "({ item }) => { item.name = item.name.replace('Name ', ''); item.extra = true; return item; }"}
Your generated dataset is now:
[{"name": "1","anotherValue": 1,"extra": true,},{"name": "2","anotherValue": 2,"extra": true,}]
filterMap and customOperationSetup
The filterMap
parameter exists to do even more complex checks. filterMap
is run in a limited context, and those are the variables available inside your function:
sift
: the sift module, so you can create a filter on-the-flyconsole.log
: tied to the 'outside'console.log
and outputs information to the actor logitem
: the current dataset itemindex
: the current filtered indextotal
: total items available in the datasetfilter
: the created filter fromquery
parameterdatasetIndex
: the current position in the dataset index
The customOperationSetup
is mostly useful to prepare a custom operation using sift
:
() => ({$gtDate(params, ownerQuery, options) {const timestamp = new Date(params).getTime();return createEqualsOperation(value => new Date(value).getTime() > timestamp, // 'value' here is the date from the field you provideownerQuery,options);}})
then use directly inside your query
("2020-01-01" is passed as param to params
):
{"query": {"lastModified": { "$gtDate": "2020-01-01" }}}
Most of the time, you won't need to use customOperationSetup
, since the built-in operators can do a lot by themselves, but they are provided for completeness.
Expected Comsumption
The memory requirements should be really low, but you need at least 128MB, the dataset items aren't loaded all at once in memory, but depending on the shape of your query, you may need more. The more query parameters you provide, more memory and CPU are required, subsequently your query finishes faster.
Limitations
Some types aren't allowed in JSON, such as Date
and RegExp
. The workaround is to define a query without those types, then inside the filterMap
, you return either null or undefined for dates or RegExp that don't match.
E.g.:
{"datasetId": "YOUR_DATASET_ID","query": {},"filterMap": "({ item }) => { if (new Date(item.someDateField).getTime() < new Date(2019, 10, 20)) { return item } }"}
Or you can use the customOperationSetup
and provide your advanced operator for native types.
License
Apache-2.0
On this page
Share Actor: