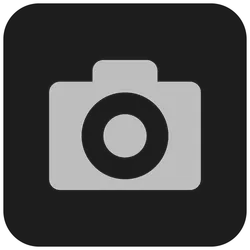
Screenshot Taker
Pricing
Pay per usage
Go to Store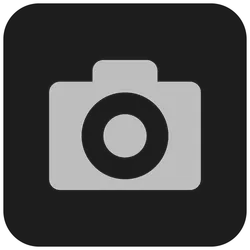
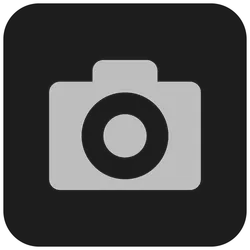
Screenshot Taker
Takes a screenshot of one or more web pages using the Chrome browser. The actor enables the setting of custom viewport size, page load timeout, delay, proxies, and output image format.
0.0 (0)
Pricing
Pay per usage
16
Total users
671
Monthly users
22
Runs succeeded
>99%
Last modified
4 years ago
Dockerfile
FROM apify/actor-node-puppeteer-chrome:16
# Second, copy just package.json since it should be the only file# that affects NPM install in the next stepCOPY package.json ./
# Install NPM packages, skip optional and development dependencies to# keep the image small. Avoid logging too much and print the dependency# tree for debuggingRUN npm --quiet set progress=false \ && npm install --only=prod --no-optional \ && echo "Installed NPM packages:" \ && (npm list || true) \ && echo "Node.js version:" \ && node --version \ && echo "NPM version:" \ && npm --version
# Next, copy the remaining files and directories with the source code.# Since we do this after NPM install, quick build will be really fast# for most source file changes.COPY . ./
INPUT_SCHEMA.json
{ "title": "Schema for the actor", "type": "object", "schemaVersion": 1, "properties": { "urls": { "title": "Page URLs", "type": "array", "description": "List of URLs of web pages to take the screenshot of.", "prefill": [ { "url": "https://www.example.com" }, { "url": "https://sdk.apify.com" } ], "editor": "requestListSources" }, "pageLoadTimeoutSecs": { "title": "Page load timeout", "type": "integer", "description": "Timeout for the web page load, in seconds. If the web page does not load in this time frame, it is considered to have failed and will be retried, similarly as with other page load errors.", "minimum": 1, "maximum": 180, "default": 60, "unit": "seconds" }, "pageMaxRetryCount": { "title": "Page retry count", "type": "integer", "description": "How many times to retry to load the page on error or timeout.", "minimum": 0, "maximum": 10, "default": 2 }, "waitUntil": { "title": "Wait until", "type": "string", "description": "Indicates when to consider the navigation to the page as succeeded. For more details, see <code>waitUntil</code> parameter of <a href='https://github.com/GoogleChrome/puppeteer/blob/master/docs/api.md#pagegotourl-options' target='_blank' rel='noopener'>Page.goto()</a> function in Puppeteer documention.", "default": "load", "enum": [ "load", "domcontentloaded", "networkidle0", "networkidle2" ], "enumTitles": [ "The load event is fired (load)", "The DOMContentLoaded event is fired (domcontentloaded)", "There are no more than 0 network connections for at least 500 ms (networkidle0)", "There are no more than 2 network connections for at least 500 ms (networkidle2)" ], "editor": "select" }, "viewportWidth": { "title": "Viewport width", "type": "integer", "description": "Width of the browser window.", "default": 1200, "minimum": 1, "maximum": 10000, "unit": "pixels" }, "viewportHeight": { "title": "Viewport height", "type": "integer", "description": "Height of the browser window.", "default": 900, "minimum": 1, "maximum": 10000, "unit": "pixels" }, "delaySecs": { "title": "Delay before screenshot", "type": "integer", "description": "How long time to wait after loading the page before taking the screenshot.", "default": 0, "minimum": 0, "maximum": 120, "unit": "seconds" }, "imageType": { "title": "Image type", "type": "string", "description": "Format of the image.", "default": "jpeg", "enum": [ "jpeg", "png" ], "enumTitles": [ "JPEG", "PNG" ], "editor": "select" }, "proxyConfiguration": { "title": "Proxy configuration", "type": "object", "description": "Specifies the type of proxy servers that will be used by the crawler in order to hide its origin.", "editor": "proxy" } }, "required": [ "urls" ]}
main.js
1const _ = require('underscore');2const Apify = require('apify');3
4Apify.main(async () => {5 const input = await Apify.getInput();6
7 const requestList = await Apify.openRequestList('my-urls', input.urls);8 const keyValueStore = await Apify.openKeyValueStore();9
10 const proxyConfiguration = await Apify.createProxyConfiguration()11
12 const crawler = new Apify.BasicCrawler({13 requestList,14 handleRequestTimeoutSecs: 120,15 maxRequestRetries: input.pageMaxRetryCount,16
17 handleRequestFunction: async ({ request }) => {18 19 // BEFORE PAGE IS NAVIGATED TO20 // Create browser instance with or without userAgent or proxy set.21 const browser = await Apify.launchPuppeteer({22 proxyUrl: proxyConfiguration.newUrl(),23 useChrome: true,24 stealth: true,25 });26 const page = await browser.newPage();27
28 if (input.viewportWidth || input.viewportHeight) {29 log(request, `Setting page viewport to ${input.viewportWidth}x${input.viewportHeight}`);30 await page.setViewport({31 width: input.viewportWidth,32 height: input.viewportHeight33 });34 }35
36 log(request, 'Loading page');37 const response = await page.goto(request.url, {38 timeout: input.pageLoadTimeoutSecs * 1000,39 });40 request.response = {41 status: response.status(),42 headers: response.headers(),43 };44
45 // Wait (if requested)46 if (input.delaySecs > 0) {47 await new Promise(resolve => setTimeout(resolve, input.delaySecs * 1000));48 }49
50 log(request, `Taking screenshot`);51 const buffer = await page.screenshot({52 type: input.imageType,53 fullPage: true,54 });55
56 // Save screenshot to key-value store57 const key = `screenshot-${Math.floor(Math.random()*0xFFFFFFFF).toString(16)}.${input.imageType}`;58 await keyValueStore.setValue(key, buffer, {59 contentType: `image/${input.imageType}`,60 });61
62 // Save record to dataset63 await Apify.pushData({64 request: _.pick(request, 'url', 'method', 'payload', 'userData'),65 response: {66 status: response.status(),67 headers: response.headers(),68 },69 finishedAt: new Date(),70 screenshot: {71 url: keyValueStore.getPublicUrl(key),72 size: buffer.length,73 },74 });75
76 log(request, 'Handling of page succeeded');77
78 try {79 await browser.close();80 } catch (e) {81 // This one can be ignored82 console.error(e);83 }84 },85
86 // This function is called if the page processing failed more than maxRequestRetries+1 times.87 handleFailedRequestFunction: async ({ request }) => {88 log(request, 'Handling of page failed');89 await Apify.pushData({90 request: _.pick(request, 'url', 'method', 'payload', 'userData'),91 response: request.response || null,92 finishedAt: new Date(), 93 errorMessages: request.errorMessages,94 });95 },96 });97
98 // Run the crawler and wait for it to finish.99 await crawler.run();100
101 console.log('Crawler finished.');102});103
104function log(request, message) {105 console.log(`[${request.url}] ${message}`);106}
package.json
{ "name": "my-actor", "version": "0.0.1", "dependencies": { "apify": "^2.0.0", "puppeteer": "*" }, "scripts": { "start": "node main.js" }, "author": "Me!"}