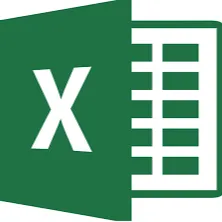
Json To Xlsx
Deprecated
Pricing
Pay per usage
Go to Store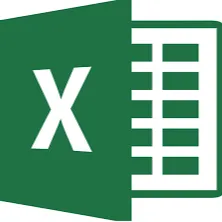
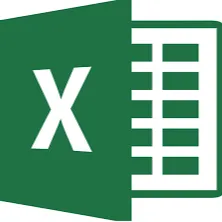
Json To Xlsx
Deprecated
petr_cermak/json-to-xlsx
The act takes a JSON input and converts it to XLSX.
0.0 (0)
Pricing
Pay per usage
7
Monthly users
2
Last modified
3 years ago
Dockerfile
1# This is a template for a Dockerfile used to run acts in Actor system.
2# The base image name below is set during the act build, based on user settings.
3# IMPORTANT: The base image must set a correct working directory, such as /usr/src/app or /home/user
4FROM apify/actor-node
5
6# Second, copy just package.json and package-lock.json since it should be
7# the only file that affects "npm install" in the next step, to speed up the build
8COPY package*.json ./
9
10# Install NPM packages, skip optional and development dependencies to
11# keep the image small. Avoid logging too much and print the dependency
12# tree for debugging
13RUN npm --quiet set progress=false \
14 && npm install --only=prod --no-optional \
15 && echo "Installed NPM packages:" \
16 && (npm list --all || true) \
17 && echo "Node.js version:" \
18 && node --version \
19 && echo "NPM version:" \
20 && npm --version
21
22# Copy source code to container
23# Do this in the last step, to have fast build if only the source code changed
24COPY . ./
25
26# NOTE: The CMD is already defined by the base image.
27# Uncomment this for local node inspector debugging:
28# CMD [ "node", "--inspect=0.0.0.0:9229", "main.js" ]
main.js
1const XLSX = require('xlsx');
2const Apify = require('apify');
3
4function toXlsxBuffer(array){
5 //flattenRecords(array)
6 const ws = XLSX.utils.json_to_sheet(array);
7 const wb = {SheetNames:['results'], Sheets:{'results': ws}};
8 const wopts = {bookType:'xlsx', bookSST:false, type:'binary'};
9 const wbout = XLSX.write(wb, wopts);
10
11 function s2ab(s){
12 const buf = new ArrayBuffer(s.length);
13 const view = new Uint8Array(buf);
14 for(let i=0; i!=s.length; ++i){
15 view[i] = s.charCodeAt(i) & 0xFF;
16 }
17 return Buffer.from(buf);
18 }
19
20 return s2ab(wbout);
21}
22
23function flattenRecord(record){
24 if(record && typeof record === 'object'){
25 for(const key in record){
26 const value = record[key];
27 if(value && Array.isArray(value)){
28 if(value.length > 0){
29 record[key] = undefined;
30 //delete record[key];
31 flattenRecords(value);
32 for(let i = 0; i < value.length; i++){
33 record[key + '/' + i] = value[i];
34 }
35 }
36 }
37 }
38 }
39}
40
41function flattenRecords(data){
42 for(let i = 0; i < data.length; i++){
43 flattenRecord(data[i]);
44 }
45}
46
47Apify.main(async () => {
48 const input = await Apify.getValue('INPUT');
49 let buffer;
50
51 if(Array.isArray(input)){
52 console.log(`Parsing a raw data array`)
53 buffer = toXlsxBuffer(input);
54 }
55 else if(input.storeId && input.key){
56 const jsonFromStore = await Apify.newClient().keyValueStore(input.storeId)
57 .getRecord(input.key)
58 .then((res) => res.value);
59 buffer = toXlsxBuffer(jsonFromStore);
60 }
61 else{
62 throw new Error('Invalid input');
63 }
64
65 const storeId = Apify.getEnv().defaultKeyValueStoreId;
66 const url = "https://api.apifier.com/v2/key-value-stores/" + storeId + "/records/results.xlsx?rawBody=1&disableRedirect=1";
67 const type = "application/octet-stream";
68 await Apify.setValue('results.xlsx', buffer, {contentType: type});
69 await Apify.setValue('OUTPUT', {output: url});
70 console.log('Output URL: ' + url);
71});
package.json
1{
2 "name": "apify-project",
3 "version": "0.0.1",
4 "description": "",
5 "author": "It's not you it's me",
6 "license": "ISC",
7 "dependencies": {
8 "xlsx": "latest",
9 "apify": "^2.2.2"
10 },
11 "scripts": {
12 "start": "node main.js"
13 }
14}
Pricing
Pricing model
Pay per usageThis Actor is paid per platform usage. The Actor is free to use, and you only pay for the Apify platform usage.