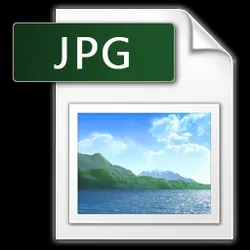
Dataset Image Downloader & Uploader
No credit card required
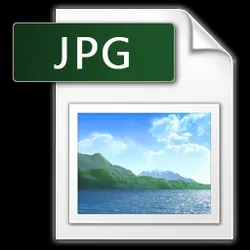
Dataset Image Downloader & Uploader
No credit card required
Download image files from image URLs in your datasets and save them to a Zip file, Key-Value store, or directly your AWS S3 bucket.
Do you want to learn more about this Actor?
Get a demoYou can access the Dataset Image Downloader & Uploader programmatically from your own applications by using the Apify API. You can choose the language preference from below. To use the Apify API, you’ll need an Apify account and your API token, found in Integrations settings in Apify Console.
1# Set API token
2API_TOKEN=<YOUR_API_TOKEN>
3
4# Prepare Actor input
5cat > input.json << 'EOF'
6{
7 "pathToImageUrls": "images",
8 "fileNameFunction": "({url, md5}) => md5(url)",
9 "uploadTo": "zip-file",
10 "preDownloadFunction": "/* Example: We get rid of the items with price 0\n({ data }) => data.filter((item) => item.price > 0)\n*/",
11 "postDownloadFunction": "/* Example: We remove items without any successfully uploaded images.\n We also remove any image URLs that were not uploaded\n \n ({ data, state }) => {\n return data.reduce((newData, item) => {\n const downloadedImages = item.images.filter((imageUrl) => {\n return state[imageUrl] && state[imageUrl].imageUploaded;\n });\n \n if (downloadedImages.length === 0) {\n return newData;\n }\n \n return newData.concat({ ...item, images: downloadedImages });\n }, []);\n}\n*/",
12 "imageCheckType": "content-type",
13 "proxyConfiguration": {
14 "useApifyProxy": true
15 }
16}
17EOF
18
19# Run the Actor using an HTTP API
20# See the full API reference at https://docs.apify.com/api/v2
21curl "https://api.apify.com/v2/acts/lukaskrivka~images-download-upload/runs?token=$API_TOKEN" \
22 -X POST \
23 -d @input.json \
24 -H 'Content-Type: application/json'
Dataset Image Downloader & Uploader API
Below, you can find a list of relevant HTTP API endpoints for calling the Dataset Image Downloader & Uploader Actor. For this, you’ll need an Apify account. Replace <YOUR_API_TOKEN> in the URLs with your Apify API token, which you can find under Integrations in Apify Console. For details, see the API reference .
Run Actor
https://api.apify.com/v2/acts/lukaskrivka~images-download-upload/runs?token=<YOUR_API_TOKEN>
Note: By adding the method=POST
query parameter, this API endpoint can be called using a GET request and thus used in third-party webhooks. Please refer to our Run Actor API documentation .
Run Actor synchronously and get dataset items
https://api.apify.com/v2/acts/lukaskrivka~images-download-upload/run-sync-get-dataset-items?token=<YOUR_API_TOKEN>
Note: This endpoint supports both POST and GET request methods. However, only the POST method allows you to pass input data. For more information, please refer to our Run Actor synchronously and get dataset items API documentation .
Get Actor
https://api.apify.com/v2/acts/lukaskrivka~images-download-upload?token=<YOUR_API_TOKEN>
For more information, please refer to our Get Actor API documentation .
Actors can be used to scrape web pages, extract data, or automate browser tasks. Use the Dataset Image Downloader & Uploader API programmatically via the Apify API.
You can choose from:
You can start Dataset Image Downloader & Uploader with the Apify API by sending an HTTP POST request to the Run Actor endpoint. An Actor’s input and its content type can be passed as a payload of the POST request, and additional options can be specified using URL query parameters. The Dataset Image Downloader & Uploader is identified within the API by its ID, which is the creator’s username and the name of the Actor.
When the Dataset Image Downloader & Uploader run finishes you can list the data from its default dataset (storage) via the API or you can preview the data directly on Apify Console .
Actor Metrics
36 monthly users
-
13 stars
>99% runs succeeded
2 days response time
Created in Nov 2018
Modified 23 days ago