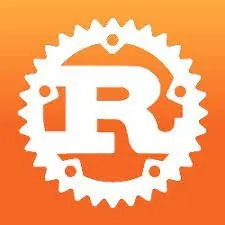
Rust Input Function Example
Try for free
No credit card required
Go to Store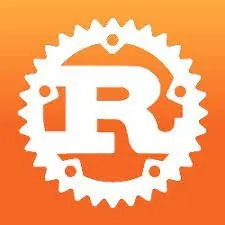
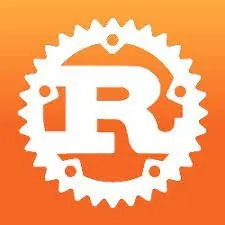
Rust Input Function Example
lukaskrivka/rust-input-function-example
Try for free
No credit card required
Dynamically compile and run input-provided page function. Like Cheerio Scraper but in Rust.
Example actor showcasing running a user-provided function in a static-typed compiled language.
How does it work
- Reads the input from disk or via Apify API
- Extracts the
page_function
string from the input - Stores the
page_function
string to the disk - Spawns a system process using
cargo
to compile thepage_function
into a dynamic library - Dynamically links the library and converts the
page_function
into a regular Rust function. It must adhere to predefined input/output types. - The example code gets HTML from the input provided
url
and parses it into adocument
using the Scraper library - The user-provided
page_function
gets thedocument
as an input parameter and returns a JSON Value type using thejson
macro
Page function
Page function can use a predefined set of Rust libraries, currently only the Scraper library and serde_json for JSON Value
type are provided.
TODO
But technically, thanks to dynamic compiling, we can enable users to provide a list of libraries to be used in the page_function
.
Example page_function
1use serde_json::{Value,json}; 2use scraper::{Html, Selector}; 3 4fn selector_to_text(document: &Html, selector: &str) -> Option<String> { 5 document 6 .select(&Selector::parse(selector).unwrap()) 7 .next() 8 .map(|el| el.text().next().unwrap().into() ) 9} 10 11#[no_mangle] 12pub fn page_function (document: &Html) -> Value { 13 println!("page_function starting"); 14 15 let title = selector_to_text(&document, "title"); 16 println!("extracted title: {:?}", title); 17 18 let header = selector_to_text(&document, "h1"); 19 println!("extracted header: {:?}", header); 20 21 let companies_using_apify = document 22 .select(&Selector::parse(".Logos__container").unwrap()) 23 .next().unwrap() 24 .select(&Selector::parse("img").unwrap()) 25 .map(|el| el.value().attr("alt").unwrap().to_string()) 26 .collect::<Vec<String>>(); 27 28 println!("extracted companies_using_apify: {:?}", companies_using_apify); 29 30 let output = json!({ 31 "title": title, 32 "header": header, 33 "companies_using_apify": companies_using_apify, 34 }); 35 println!("inside pageFunction output: {:?}", output); 36 output 37}
Developer
Maintained by Community
Actor Metrics
1 monthly user
-
2 stars
Created in Dec 2023
Modified 2 months ago
Categories