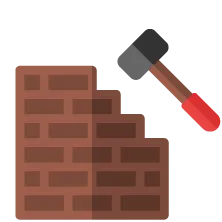
Rebuilder
Pricing
Pay per usage
Go to Store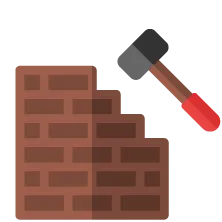
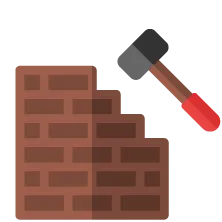
Rebuilder
Rebuild your actors easily with a simple regular expression. This actor will fetch all your existing actors and match their names. Those that pass will be rebuilt. Schedule this for maximum effectiveness. It can also rebuild itself!
0.0 (0)
Pricing
Pay per usage
1
Total users
2
Monthly users
1
Runs succeeded
>99%
Last modified
3 years ago
Dockerfile
FROM apify/actor-node-basic:beta# Copy source codeCOPY . ./#COPY src ./srcRUN npm --quiet set progress=false \&& npm install --only=prod --no-optional \&& echo "Installed NPM packages:" \&& npm list || true \&& echo "Node.js version:" \&& node --version \&& echo "NPM version:" \&& npm --version
INPUT_SCHEMA.json
{ "title": "Rebuilder Input", "type": "object", "description": "Rebuild your actors easily with a simple regular expression. This actor will fetch all your existing actors and match their names. Those that pass will be rebuilt. Schedule this for maximum effectiveness. It can also rebuild itself!", "schemaVersion": 1, "properties": { "regexString": { "title": "Regular Expression", "type": "string", "description": "Regular expression to match the name of the actors you want to rebuild. It's a string that will be used to construct a RegExp instance, so make sure to use proper escaping!", "editor": "textfield" }, "version": { "title": "Version", "type": "string", "description": "Other versions than 0.0 of the actors can be rebuilt if a different version is specified. Unfortunately, using tags is not supported by Apify at the moment.", "editor": "textfield", "default": "0.0" }, "buildTag": { "title": "Build tag", "type": "string", "description": "Tag to use for all the builds.", "editor": "textfield", "default": "latest" }, "waitForFinish": { "title": "Wait for finish", "type": "boolean", "description": "Check this to tell the actor to wait for completion of all builds. This is useful when tied with a webhook that would then notify you of errors.", "default": false } }, "required": ["regexString"]}
main.js
1const Apify = require('apify');2
3const { utils: { log, sleep }, client } = Apify;4
5Apify.main(async () => {6 log.info('Getting input.');7 const {8 regexString,9 waitForFinish,10 version,11 buildTag12 } = await Apify.getInput();13
14 log.info('Constructing regex.')15 const regex = new RegExp(regexString, 'i');16
17 log.info('Getting all user\'s actors.');18 const { items } = await client.acts.listActs();19
20 log.info('Filtering items that match our regex.');21 const actorsToRebuild = items.filter(({ name }) => regex.test(name));22 const actorCount = actorsToRebuild.length;23 if (actorCount > 50) {24 throw new Error(`Too many actors to rebuild (${actorCount}).`25 + 'Max is 50. Use a more specific regular expression.');26 }27
28 log.info(`Starting build of ${actorCount} actors.`);29 let failedBuildsCount = 0;30 const promises = actorsToRebuild.map(async ({ id }, idx) => {31 await sleep(idx * 500);32 return client.acts.buildAct({33 actId: id,34 version,35 useCache: false,36 tag: buildTag,37 waitForFinish: waitForFinish ? 120 : 038 }).catch(err => {39 log.exception(err);40 failedBuildsCount++41 });42 43 });44 await Promise.all(promises);45
46 log.info(`All builds ${waitForFinish ? 'finished' : 'dispatched'}.`);47 if (failedBuildsCount) {48 throw new Error('Some of the builds were not successful. See log.');49 }50});
package.json
{ "name": "rebuilder", "version": "0.0.1", "description": "Actors description", "main": "main.js", "dependencies": { "apify": "beta" }, "scripts": { "start": "node main.js" }, "author": "mnmkng"}