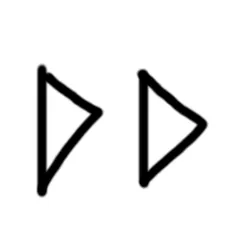
Forward Dataset to Actor or Task
Pricing
Pay per usage
Go to Store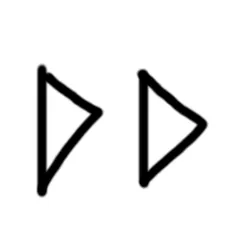
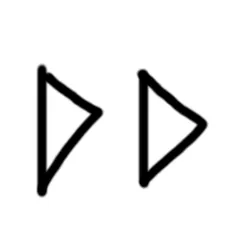
Forward Dataset to Actor or Task
Forwards contents of specified dataset to a specified field on the input of another Actor or task.
0.0 (0)
Pricing
Pay per usage
6
Total users
7
Monthly users
1
Runs succeeded
0%
Last modified
2 years ago
.actor/Dockerfile
# Specify the base Docker imageFROM oven/bun:1.0.7
# Next, copy the source files using the user set# in the base image.COPY . ./
# Install all dependencies. RUN bun install
# Run the image.CMD bun run start
.actor/actor.json
{ "actorSpecification": 1, "name": "forward-dataset-to-actor-or-task", "title": "Forward dataset to Actor or task", "description": "Forwards specified field of dataset to Actor or task", "version": "0.0", "meta": { "templateId": "ts-start-bun" }, "input": "./input_schema.json", "dockerfile": "./Dockerfile"}
.actor/input_schema.json
{ "title": "Scrape data from a web page", "type": "object", "schemaVersion": 1, "properties": { "datasetId": { "sectionCaption": "Source dataset", "title": "Dataset ID", "type": "string", "description": "Id of dataset that should be forwarded", "editor": "textfield" }, "datasetFieldName": { "title": "Dataset field name", "type": "string", "description": "Name of the dataset field", "editor": "textfield" }, "targetType": { "sectionCaption": "Target", "title": "Type", "type": "string", "description": "Select if the target is Actor or task", "editor": "select", "enum": ["ACTOR", "TASK"], "enumTitles": ["Actor", "Task"], "prefill": "TASK" }, "targetId": { "title": "Target ID or name", "type": "string", "description": "", "editor": "textfield" }, "targetFieldName": { "title": "Field name", "type": "string", "description": "Name of the field on input of target Actor or task that the dataset should be mapped to.", "editor": "textfield" }, "format": { "title": "Format", "description": "Pick the format that should be used. Corresponds to target field format.", "type": "string", "editor": "select", "enum": ["stringList", "requestListSources"], "enumTitles": ["String list", "Request list sources"], "prefill": "stringList" }, "inputOverride": { "title": "Input override", "type": "object", "description": "Input override", "editor": "json" }, "optionsOverride": { "title": "Options override", "type": "object", "description": "Options override", "editor": "json" } }, "required": ["datasetId", "datasetFieldName", "format", "targetFieldName", "targetType", "targetId"]}
src/main.ts
1import { Actor, log } from 'apify';2
3await Actor.init();4
5interface Input {6 datasetId: string;7 datasetFieldName: string;8 format: "stringList" | "requestListSources",9 targetType: "ACTOR" | "TASK",10 targetId: string;11 targetFieldName: string;12 inputOverride: object;13 optionsOverride: object;14}15
16const input = await Actor.getInput<Input>();17if (!input) throw new Error("Input is missing!");18
19const { 20 datasetId,21 datasetFieldName,22 format,23 targetType,24 targetId,25 targetFieldName,26 inputOverride = {},27 optionsOverride = {},28 } = input;29
30const { apifyClient } = Actor;31
32// Get Actor or task client33const targetClient = targetType === 'TASK' ? apifyClient.task(targetId) : apifyClient.actor(targetId);34// Check if the target actually exists35if (!await targetClient.get()) {36 await Actor.fail(`The ${targetType} "${targetId}" was not found.`);37}38
39// Prepare a format function, that will be applied to each item40const formatFunction = format === 'requestListSources' ? (url) => ({url}) : (value) => value;41
42
43// The variable that will contain the specified field from all items in dataset.44const datasetAsArray = [];45
46await Actor.setStatusMessage('Loading data from dataset...');47
48// Loop over all entries in dataset, in batches.49let offset = 0, total = 0, items = [];50do {51 const response = await apifyClient.dataset(datasetId).listItems({52 fields: [ datasetFieldName ],53 offset,54 limit: 200,55 });56 items = response.items;57 total = response.total;58 offset += items.length;59
60 items.forEach(sourceItem => {61 // Format the item to desired shape62 const targetItem = formatFunction(sourceItem[datasetFieldName]);63 // Push it to the target array64 datasetAsArray.push(targetItem);65 });66 67 await Actor.setStatusMessage(`Loaded ${datasetAsArray.length}/${total} dataset items.`);68} while(items.length > 0);69
70// Update the field in input71inputOverride[targetFieldName] = datasetAsArray;72
73await Actor.setStatusMessage(`Dataset loaded, starting target ${targetType} ${targetId}`);74
75// Start the target with specified input and options, don't wait for finish.76await targetClient.start(inputOverride, { ...optionsOverride, waitForFinish: 0});77
78await Actor.exit();
.dockerignore
# configurations.idea
# crawlee and apify storage foldersapify_storagecrawlee_storagestorage
# installed filesnode_modules
# git folder.git
# dist folderdist
.gitignore
storageapify_storagecrawlee_storagenode_modulesdisttsconfig.tsbuildinfostorage/*!storage/key_value_storesstorage/key_value_stores/*!storage/key_value_stores/defaultstorage/key_value_stores/default/*!storage/key_value_stores/default/INPUT.json
package.json
{ "name": "ts-start-bun", "version": "0.0.1", "type": "module", "description": "This is an example of an Apify actor.", "engines": { "bun": ">=1.0.0" }, "dependencies": { "apify": "^3.1.10" }, "devDependencies": { "@apify/tsconfig": "^0.1.0" }, "scripts": { "start": "bun src/main.ts", "test": "echo \"Error: oops, the actor has no tests yet, sad!\" && exit 1" }, "author": "It's not you it's me", "license": "ISC"}
tsconfig.json
{ "extends": "@apify/tsconfig", "compilerOptions": { "module": "ES2022", "target": "ES2022", "outDir": "dist", "noUnusedLocals": false, "lib": ["DOM"] }, "include": [ "./src/**/*" ]}